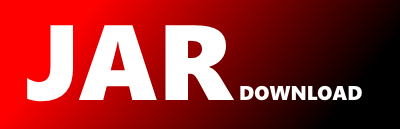
com.quotemedia.streamer.client.mapper.PriceDataMsgImpl Maven / Gradle / Ivy
package com.quotemedia.streamer.client.mapper;
import com.quotemedia.datacache.data.Price;
import com.quotemedia.datacache.data.QMDecimal;
import com.quotemedia.datacache.data.QMLongDecimal;
import com.quotemedia.streamer.messages.market.DataMessage;
import java.util.Date;
public class PriceDataMsgImpl implements DataMessage {
private String symbol;
private int locateCode;
private Date timestamp;
private Price accumulatedPrice;
private Price accumulatedTradeValue;
private QMLongDecimal accumulatedVolume;
private Price change;
private Price close;
private Price high;
private Price last;
private QMDecimal lastTradeSize;
private Date lastTradeTime;
private Price low;
private Price open;
private Float percentChange;
private Price previousClose;
private Tick tick;
private Long tradeCount;
private Price TWAP;
private Price VWAP;
private QMLongDecimal vwapVolume;
private Boolean annualHigh;
private Boolean annualLow;
private Date preMarketTradeTime;
private Price preMarketLast;
private QMLongDecimal preMarketVolume;
private Price preMarketChange;
private Float preMarketPercentChange;
private Date postMarketTradeTime;
private Price postMarketLast;
private QMLongDecimal postMarketVolume;
private Price postMarketChange;
private Float postMarketPercentChange;
private String lastTradeExcode;
private String currencyID;
public String getSymbol() {
return symbol;
}
public void setSymbol(String symbol) {
this.symbol = symbol;
}
public int getLocateCode() {
return locateCode;
}
public void setLocateCode(int locateCode) {
this.locateCode = locateCode;
}
public Date getTimestamp() {
return timestamp;
}
public void setTimestamp(Date timestamp) {
this.timestamp = timestamp;
}
public Price getAccumulatedPrice() {
return accumulatedPrice;
}
public void setAccumulatedPrice(Price accumulatedPrice) {
this.accumulatedPrice = accumulatedPrice;
}
public Price getAccumulatedTradeValue() {
return accumulatedTradeValue;
}
public void setAccumulatedTradeValue(Price accumulatedTradeValue) {
this.accumulatedTradeValue = accumulatedTradeValue;
}
public QMLongDecimal getAccumulatedVolume() {
return accumulatedVolume;
}
public void setAccumulatedVolume(QMLongDecimal accumulatedVolume) {
this.accumulatedVolume = accumulatedVolume;
}
public Price getChange() {
return change;
}
public void setChange(Price change) {
this.change = change;
}
public Price getClose() {
return close;
}
public void setClose(Price close) {
this.close = close;
}
public Price getHigh() {
return high;
}
public void setHigh(Price high) {
this.high = high;
}
public Price getLast() {
return last;
}
public void setLast(Price last) {
this.last = last;
}
public QMDecimal getLastTradeSize() {
return lastTradeSize;
}
public void setLastTradeSize(QMDecimal lastTradeSize) {
this.lastTradeSize = lastTradeSize;
}
public Date getLastTradeTime() {
return lastTradeTime;
}
public void setLastTradeTime(Date lastTradeTime) {
this.lastTradeTime = lastTradeTime;
}
public Price getLow() {
return low;
}
public void setLow(Price low) {
this.low = low;
}
public Price getOpen() {
return open;
}
public void setOpen(Price open) {
this.open = open;
}
public Float getPercentChange() {
return percentChange;
}
public void setPercentChange(Float percentChange) {
this.percentChange = percentChange;
}
public Price getPreviousClose() {
return previousClose;
}
public void setPreviousClose(Price previousClose) {
this.previousClose = previousClose;
}
public Tick getTick() {
return tick;
}
public void setTick(Tick tick) {
this.tick = tick;
}
public Long getTradeCount() {
return tradeCount;
}
public void setTradeCount(Long tradeCount) {
this.tradeCount = tradeCount;
}
public Price getTWAP() {
return TWAP;
}
public void setTWAP(Price TWAP) {
this.TWAP = TWAP;
}
public Price getVWAP() {
return VWAP;
}
public void setVWAP(Price VWAP) {
this.VWAP = VWAP;
}
public Boolean getAnnualHigh() {
return annualHigh;
}
public void setAnnualHigh(Boolean annualHigh) {
this.annualHigh = annualHigh;
}
public Boolean getAnnualLow() {
return annualLow;
}
public void setAnnualLow(Boolean annualLow) {
this.annualLow = annualLow;
}
public Date getPreMarketTradeTime() {
return preMarketTradeTime;
}
public void setPreMarketTradeTime(Date preMarketTradeTime) {
this.preMarketTradeTime = preMarketTradeTime;
}
public Price getPreMarketLast() {
return preMarketLast;
}
public void setPreMarketLast(Price preMarketLast) {
this.preMarketLast = preMarketLast;
}
public QMLongDecimal getPreMarketVolume() {
return preMarketVolume;
}
public void setPreMarketVolume(QMLongDecimal preMarketVolume) {
this.preMarketVolume = preMarketVolume;
}
public Price getPreMarketChange() {
return preMarketChange;
}
public void setPreMarketChange(Price preMarketChange) {
this.preMarketChange = preMarketChange;
}
public Float getPreMarketPercentChange() {
return preMarketPercentChange;
}
public void setPreMarketPercentChange(Float preMarketPercentChange) {
this.preMarketPercentChange = preMarketPercentChange;
}
public Date getPostMarketTradeTime() {
return postMarketTradeTime;
}
public void setPostMarketTradeTime(Date postMarketTradeTime) {
this.postMarketTradeTime = postMarketTradeTime;
}
public Price getPostMarketLast() {
return postMarketLast;
}
public void setPostMarketLast(Price postMarketLast) {
this.postMarketLast = postMarketLast;
}
public QMLongDecimal getPostMarketVolume() {
return postMarketVolume;
}
public void setPostMarketVolume(QMLongDecimal postMarketVolume) {
this.postMarketVolume = postMarketVolume;
}
public Price getPostMarketChange() {
return postMarketChange;
}
public void setPostMarketChange(Price postMarketChange) {
this.postMarketChange = postMarketChange;
}
public Float getPostMarketPercentChange() {
return postMarketPercentChange;
}
public void setPostMarketPercentChange(Float postMarketPercentChange) {
this.postMarketPercentChange = postMarketPercentChange;
}
public String getLastTradeExcode() {
return lastTradeExcode;
}
public void setLastTradeExcode(String lastTradeExcode) {
this.lastTradeExcode = lastTradeExcode;
}
public String getCurrencyID() {
return currencyID;
}
public void setCurrencyID(String currencyID) {
this.currencyID = currencyID;
}
public QMLongDecimal getVwapVolume() {
return vwapVolume;
}
public void setVwapVolume(QMLongDecimal vwapVolume) {
this.vwapVolume = vwapVolume;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy