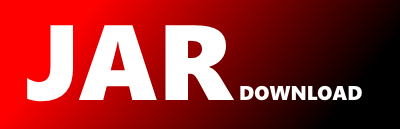
com.qwlabs.tree.Location Maven / Gradle / Ivy
package com.qwlabs.tree;
import com.google.common.collect.Lists;
import jakarta.validation.constraints.NotNull;
import lombok.Getter;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Function;
import java.util.stream.Collectors;
@Getter
public class Location {
private static final RootLocation ROOT = new RootLocation<>();
@NotNull
private final List path;
public Location(List path) {
this.path = path;
}
@NotNull
public Optional> parent() {
return isRoot() ? Optional.empty() :
Optional.of(Location.of(path.subList(0, path.size() - 1)));
}
public boolean isRoot() {
return path.isEmpty();
}
public Location map(Function mapper) {
return Location.of(mapPath(mapper));
}
public List mapPath(Function mapper) {
return path.stream().map(mapper)
.collect(Collectors.toList());
}
public Location child(N node) {
List childPath = Lists.newArrayList(path);
childPath.add(node);
return Location.of(childPath);
}
public Optional tail() {
return isRoot() ? Optional.empty() : Optional.of(path.get(path.size() - 1));
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Location> location = (Location>) o;
return Objects.equals(path, location.path);
}
@Override
public int hashCode() {
return Objects.hash(path);
}
public static Location root() {
return (Location) ROOT;
}
public static Location of(List path) {
return new Location<>(path);
}
public static Location of(N... path) {
return of(List.of(path));
}
public static class RootLocation> extends Location {
public RootLocation() {
super(List.of());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy