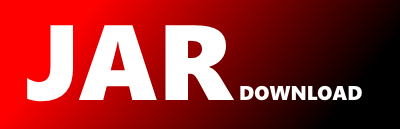
com.qwlabs.tree.TreeNodes Maven / Gradle / Ivy
package com.qwlabs.tree;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.qwlabs.lang.Streams2;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.BiPredicate;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
@JsonInclude(value = JsonInclude.Include.NON_EMPTY)
public class TreeNodes extends ArrayList> implements TreeNodeAble {
private static final TreeNodes EMPTY = new TreeNodes(0);
@JsonCreator
public TreeNodes() {
}
public TreeNodes(int initialCapacity) {
super(initialCapacity);
}
public TreeNodes(Collection extends TreeNode> c) {
super(c);
}
@Override
public void forEach(BiConsumer>, TreeNode> consumer,
Location> parentLocation,
boolean parallel) {
Streams2.parallel(this.stream(), parallel).forEach(node -> node.forEach(consumer, parentLocation));
}
@Override
public Optional> find(List path,
BiPredicate, E> filter,
boolean parallel) {
if (path.isEmpty()) {
return Optional.empty();
}
return Streams2.parallel(this.stream(), parallel)
.map(node -> node.find(path, filter))
.filter(Optional::isPresent)
.map(Optional::get)
.findFirst();
}
@Override
public Optional>> find(BiPredicate>, TreeNode> filter,
Location> parentLocation,
boolean parallel) {
return Streams2.parallel(this.stream(), parallel)
.map(node -> node.find(filter, parentLocation))
.filter(Optional::isPresent)
.map(Optional::get)
.findFirst();
}
@Override
public Stream all(BiFunction>, TreeNode, R> mapper, Location> parentLocation) {
return stream().flatMap(node -> node.all(mapper, parentLocation));
}
public TreeNodes map(BiFunction>, TreeNode, R> mapper) {
return map(mapper, Location.root(), false);
}
public TreeNodes mapParallel(BiFunction>, TreeNode, R> mapper) {
return map(mapper, Location.root(), true);
}
public TreeNodes map(BiFunction>, TreeNode, R> mapper,
boolean parallel) {
return map(mapper, Location.root(), parallel);
}
public TreeNodes map(BiFunction>, TreeNode, R> mapper,
Location> parentLocation) {
return map(mapper, parentLocation, false);
}
public TreeNodes mapParallel(BiFunction>, TreeNode, R> mapper,
Location> parentLocation) {
return map(mapper, parentLocation, true);
}
public TreeNodes map(BiFunction>, TreeNode, R> mapper,
Location> parentLocation,
boolean parallel) {
return new TreeNodes<>(Streams2.parallel(this.stream(), parallel)
.map(node -> node.map(mapper, parentLocation))
.collect(Collectors.toList()));
}
public List map(Function mapper) {
return map(mapper, false);
}
public List mapParallel(Function mapper) {
return map(mapper, true);
}
public List map(Function mapper, boolean parallel) {
return Streams2.parallel(this.stream(), parallel).map(node -> node.map(mapper)).collect(Collectors.toList());
}
public List map(TreeNodeFunction mapper) {
return map(mapper, Location.root(), false);
}
public List mapParallel(TreeNodeFunction mapper) {
return map(mapper, Location.root(), true);
}
public List map(TreeNodeFunction mapper, boolean parallel) {
return map(mapper, Location.root(), parallel);
}
public List map(TreeNodeFunction mapper,
Location> parentLocation) {
return map(mapper, parentLocation, false);
}
public List mapParallel(TreeNodeFunction mapper,
Location> parentLocation) {
return map(mapper, parentLocation, true);
}
public List map(TreeNodeFunction mapper,
Location> parentLocation,
boolean parallel) {
return Streams2.parallel(this.stream(), parallel)
.map(node -> node.map(mapper, parentLocation, parallel)).collect(Collectors.toList());
}
public Optional> first() {
return stream().findFirst();
}
public boolean isSingle() {
return size() == 1;
}
public boolean isMultiple() {
return size() > 1;
}
public static TreeNodes of(TreeNode... nodes) {
if (nodes == null || nodes.length == 0) {
return new TreeNodes<>();
}
return new TreeNodes<>(Arrays.asList(nodes));
}
public static TreeNodes empty() {
return (TreeNodes) EMPTY;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy