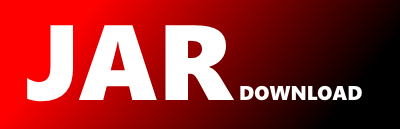
com.rabbitmq.perf.VaryingScenarioStats Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of perf-test Show documentation
Show all versions of perf-test Show documentation
A Java-based performance testing tool for RabbitMQ.
// Copyright (c) 2007-Present Pivotal Software, Inc. All rights reserved.
//
// This software, the RabbitMQ Java client library, is triple-licensed under the
// Mozilla Public License 1.1 ("MPL"), the GNU General Public License version 2
// ("GPL") and the Apache License version 2 ("ASL"). For the MPL, please see
// LICENSE-MPL-RabbitMQ. For the GPL, please see LICENSE-GPL2. For the ASL,
// please see LICENSE-APACHE2.
//
// This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY KIND,
// either express or implied. See the LICENSE file for specific language governing
// rights and limitations of this software.
//
// If you have any questions regarding licensing, please contact us at
// [email protected].
package com.rabbitmq.perf;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class VaryingScenarioStats implements ScenarioStats {
private final Map, SimpleScenarioStats> stats = new HashMap, SimpleScenarioStats>();
private final List> keys = new ArrayList>();
public VaryingScenarioStats() {}
public SimpleScenarioStats next(List value) {
SimpleScenarioStats stats = new SimpleScenarioStats(1000L);
keys.add(value);
this.stats.put(value, stats);
return stats;
}
@SuppressWarnings("unchecked")
public Map results() {
Map map = new HashMap();
List dimensions = new ArrayList();
for (VariableValue keyElem : keys.get(0)) {
dimensions.add(keyElem.getName());
}
map.put("dimensions", dimensions);
Map> dimensionValues = new HashMap>();
for (List key : keys) {
for (VariableValue elem : key) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy