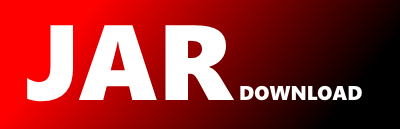
com.rabobank.argos.argos4j.rest.api.client.LinkApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of argos4j Show documentation
Show all versions of argos4j Show documentation
Java client for Argos Supply Chain Notary
package com.rabobank.argos.argos4j.rest.api.client;
import com.rabobank.argos.argos4j.rest.api.ApiClient;
import com.rabobank.argos.argos4j.rest.api.EncodingUtils;
import com.rabobank.argos.argos4j.rest.api.model.RestError;
import com.rabobank.argos.argos4j.rest.api.model.RestLinkMetaBlock;
import com.rabobank.argos.argos4j.rest.api.model.RestValidationError;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import feign.*;
public interface LinkApi extends ApiClient.Api {
/**
* Create a link
*
* @param supplyChainId supply chain id (required)
* @param restLinkMetaBlock (optional)
*/
@RequestLine("POST /supplychain/{supplyChainId}/link")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
void createLink(@Param("supplyChainId") String supplyChainId, RestLinkMetaBlock restLinkMetaBlock);
/**
* find link
*
* @param supplyChainId supply chain id (required)
* @param hash hash of product or material (optional)
* @return List<RestLinkMetaBlock>
*/
@RequestLine("GET /supplychain/{supplyChainId}/link?hash={hash}")
@Headers({
"Accept: application/json",
})
List findLink(@Param("supplyChainId") String supplyChainId, @Param("hash") String hash);
/**
* find link
*
* Note, this is equivalent to the other findLink
method,
* but with the query parameters collected into a single Map parameter. This
* is convenient for services with optional query parameters, especially when
* used with the {@link FindLinkQueryParams} class that allows for
* building up this map in a fluent style.
* @param supplyChainId supply chain id (required)
* @param queryParams Map of query parameters as name-value pairs
* The following elements may be specified in the query map:
*
* - hash - hash of product or material (optional)
*
* @return List<RestLinkMetaBlock>
*/
@RequestLine("GET /supplychain/{supplyChainId}/link?hash={hash}")
@Headers({
"Accept: application/json",
})
List findLink(@Param("supplyChainId") String supplyChainId, @QueryMap(encoded=true) Map queryParams);
/**
* A convenience class for generating query parameters for the
* findLink
method in a fluent style.
*/
public static class FindLinkQueryParams extends HashMap {
public FindLinkQueryParams hash(final String value) {
put("hash", EncodingUtils.encode(value));
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy