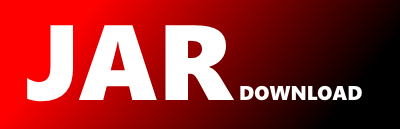
org.rajivprab.sava.keys.ApiKeysSwitch Maven / Gradle / Ivy
package org.rajivprab.sava.keys;
import com.google.common.collect.ImmutableMap;
/**
* MUXed ApiKeys that can be used to toggle between QA and prod mode behind the scenes.
*
* Merely a simplified version of ApiKeysMux, that only supports 2 environments.
* Is Thread-Safe as long as all ApiKeys provided are thread-safe.
*
* Created by rprabhakar on 2/1/16.
*/
public class ApiKeysSwitch implements ApiKeys {
private static final String PROD = "prod";
private static final String QA = "qa";
private final ApiKeysMux apiKeysMux;
// ------------------------- Constructors --------------------------
public static ApiKeysSwitch build(ApiKeys prod, ApiKeys qa) {
return new ApiKeysSwitch(prod, qa);
}
private ApiKeysSwitch(ApiKeys prod, ApiKeys qa) {
this.apiKeysMux = ApiKeysMux.build(PROD, ImmutableMap.of(PROD, prod, QA, qa));
}
// --------------------------------------------------------------
public void useQA() {
try {
apiKeysMux.setEnvironment(QA);
} catch (IllegalArgumentException e) {
if (e.getMessage().equals("New value: qa, does not match existing value: prod")) {
throw new IllegalArgumentException("Prod credentials have already been used prior to useQA");
} else {
throw e;
}
}
}
@Override
public String getCredentials(String key) {
return apiKeysMux.getCredentials(key);
}
}