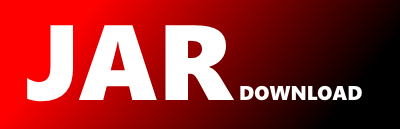
org.rajivprab.sava.logging.BufferedDispatcher Maven / Gradle / Ivy
package org.rajivprab.sava.logging;
import com.google.common.collect.HashBasedTable;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Table;
import java.util.Map;
import java.util.Set;
// Very simple implementation, suitable for testing purposes. Not thread-safe. Does not make defensive copies.
public class BufferedDispatcher implements Dispatcher {
private final Table dispatches = HashBasedTable.create();
private Set ignore = ImmutableSet.of();
public BufferedDispatcher ignore(Severity... severities) {
ignore = ImmutableSet.copyOf(severities);
return this;
}
@Override
public void dispatch(Severity severity, String title, String message) {
if (!ignore.contains(severity)) {
dispatches.put(severity, title, message);
}
}
public Map getDispatches(Severity severity) {
return dispatches.row(severity);
}
public Table getDispatches() {
return dispatches;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy