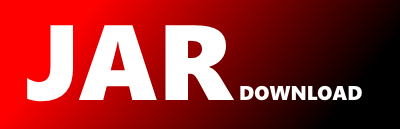
doc.com.rallydev.rest.client.HttpClient.html Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rally-rest-api Show documentation
Show all versions of rally-rest-api Show documentation
A java toolkit for interacting with the Rally Rest API
HttpClient (Rally Rest API for Java 2.1)
com.rallydev.rest.client
Class HttpClient
- java.lang.Object
-
- org.apache.http.impl.client.AbstractHttpClient
-
- org.apache.http.impl.client.DefaultHttpClient
-
- com.rallydev.rest.client.HttpClient
-
- All Implemented Interfaces:
- Closeable, AutoCloseable, HttpClient
- Direct Known Subclasses:
- ApiKeyClient, BasicAuthClient
public class HttpClient
extends DefaultHttpClient
implements Closeable
A HttpClient implementation providing connectivity to Rally. This class does not
provide any authentication on its own but instead relies on a concrete subclass to do so.
-
-
Method Summary
Methods
Modifier and Type
Method and Description
void
close()
Release all resources associated with this instance.
String
doDelete(String url)
Perform a delete against the WSAPI
String
doGet(String url)
Perform a get against the WSAPI
String
doPost(String url,
String body)
Perform a post against the WSAPI
String
doPut(String url,
String body)
Perform a put against the WSAPI
String
getServer()
Get the current server being targeted.
String
getWsapiUrl()
Get the WSAPI base url based on the current server and WSAPI version
String
getWsapiVersion()
Get the current version of the WSAPI being targeted.
void
setApplicationName(String value)
Set the value of the X-RallyIntegrationName header included on all requests.
void
setApplicationVendor(String value)
Set the value of the X-RallyIntegrationVendor header included on all requests.
void
setApplicationVersion(String value)
Set the value of the X-RallyIntegrationVersion header included on all requests.
void
setProxy(URI proxy)
Set the unauthenticated proxy server to use.
void
setProxy(URI proxy,
String userName,
String password)
Set the authenticated proxy server to use.
void
setWsapiVersion(String wsapiVersion)
Set the current version of the WSAPI being targeted.
-
Methods inherited from class org.apache.http.impl.client.DefaultHttpClient
setDefaultHttpParams
-
Methods inherited from class org.apache.http.impl.client.AbstractHttpClient
addRequestInterceptor, addRequestInterceptor, addResponseInterceptor, addResponseInterceptor, clearRequestInterceptors, clearResponseInterceptors, execute, execute, execute, execute, execute, execute, execute, execute, getAuthSchemes, getBackoffManager, getConnectionBackoffStrategy, getConnectionKeepAliveStrategy, getConnectionManager, getConnectionReuseStrategy, getCookieSpecs, getCookieStore, getCredentialsProvider, getHttpRequestRetryHandler, getParams, getProxyAuthenticationHandler, getProxyAuthenticationStrategy, getRedirectHandler, getRedirectStrategy, getRequestExecutor, getRequestInterceptor, getRequestInterceptorCount, getResponseInterceptor, getResponseInterceptorCount, getRoutePlanner, getTargetAuthenticationHandler, getTargetAuthenticationStrategy, getUserTokenHandler, removeRequestInterceptorByClass, removeResponseInterceptorByClass, setAuthSchemes, setBackoffManager, setConnectionBackoffStrategy, setCookieSpecs, setCookieStore, setCredentialsProvider, setHttpRequestRetryHandler, setKeepAliveStrategy, setParams, setProxyAuthenticationHandler, setProxyAuthenticationStrategy, setRedirectHandler, setRedirectStrategy, setReuseStrategy, setRoutePlanner, setTargetAuthenticationHandler, setTargetAuthenticationStrategy, setUserTokenHandler
-
-
Method Detail
-
setProxy
public void setProxy(URI proxy)
Set the unauthenticated proxy server to use. By default no proxy is configured.
- Parameters:
proxy
- The proxy server, e.g. new URI("http://my.proxy.com:8000")
-
setProxy
public void setProxy(URI proxy,
String userName,
String password)
Set the authenticated proxy server to use. By default no proxy is configured.
- Parameters:
proxy
- The proxy server, e.g. new URI("http://my.proxy.com:8000")
userName
- The username to be used for authentication.password
- The password to be used for authentication.
-
setApplicationVendor
public void setApplicationVendor(String value)
Set the value of the X-RallyIntegrationVendor header included on all requests.
This should be set to your company name.
- Parameters:
value
- The vendor header to be included on all requests.
-
setApplicationVersion
public void setApplicationVersion(String value)
Set the value of the X-RallyIntegrationVersion header included on all requests.
This should be set to the version of your application.
- Parameters:
value
- The vendor header to be included on all requests.
-
setApplicationName
public void setApplicationName(String value)
Set the value of the X-RallyIntegrationName header included on all requests.
This should be set to the name of your application.
- Parameters:
value
- The vendor header to be included on all requests.
-
getServer
public String getServer()
Get the current server being targeted.
- Returns:
- the current server.
-
getWsapiVersion
public String getWsapiVersion()
Get the current version of the WSAPI being targeted.
- Returns:
- the current WSAPI version.
-
setWsapiVersion
public void setWsapiVersion(String wsapiVersion)
Set the current version of the WSAPI being targeted.
- Parameters:
wsapiVersion
- the new version, e.g. "1.30"
-
doPost
public String doPost(String url,
String body)
throws IOException
Perform a post against the WSAPI
- Parameters:
url
- the request urlbody
- the body of the post
- Returns:
- the JSON encoded string response
- Throws:
IOException
- if a non-200 response code is returned or if some other
problem occurs while executing the request
-
doPut
public String doPut(String url,
String body)
throws IOException
Perform a put against the WSAPI
- Parameters:
url
- the request urlbody
- the body of the put
- Returns:
- the JSON encoded string response
- Throws:
IOException
- if a non-200 response code is returned or if some other
problem occurs while executing the request
-
doDelete
public String doDelete(String url)
throws IOException
Perform a delete against the WSAPI
- Parameters:
url
- the request url
- Returns:
- the JSON encoded string response
- Throws:
IOException
- if a non-200 response code is returned or if some other
problem occurs while executing the request
-
doGet
public String doGet(String url)
throws IOException
Perform a get against the WSAPI
- Parameters:
url
- the request url
- Returns:
- the JSON encoded string response
- Throws:
IOException
- if a non-200 response code is returned or if some other
problem occurs while executing the request
-
close
public void close()
throws IOException
Release all resources associated with this instance.
- Specified by:
close
in interface Closeable
- Specified by:
close
in interface AutoCloseable
- Throws:
IOException
- if an error occurs releasing resources
-
getWsapiUrl
public String getWsapiUrl()
Get the WSAPI base url based on the current server and WSAPI version
- Returns:
- the fully qualified WSAPI base url, e.g. https://rally1.rallydev.com/slm/webservice/1.33
© 2015 - 2025 Weber Informatics LLC | Privacy Policy