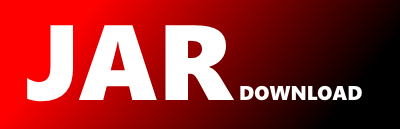
com.rapatao.vertx.eventbus.proxyhelper.ServiceRegistry Maven / Gradle / Ivy
package com.rapatao.vertx.eventbus.proxyhelper;
import io.vertx.core.eventbus.EventBus;
import io.vertx.core.logging.Logger;
import io.vertx.core.logging.LoggerFactory;
import lombok.AccessLevel;
import lombok.RequiredArgsConstructor;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
/**
* Event Bus Service Registry
*
* This class is responsibly to registry all services int the Vertx EventBus.
*
* Created by rapatao on 13/09/16
*/
@RequiredArgsConstructor(access = AccessLevel.PRIVATE)
public class ServiceRegistry {
private final static Logger logger = LoggerFactory.getLogger(ServiceRegistry.class);
private final EventBus eventBus;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy