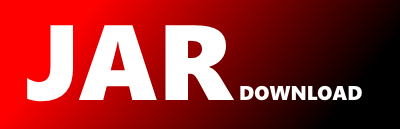
com.rathravane.drumlin.service.framework.routing.playish.DrumlinPlayishStaticEntryPointRoutingSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of drumlin Show documentation
Show all versions of drumlin Show documentation
A simple Java webapp framework with POJO path routing, Velocity templates, and basic
app functionality such as HTML form validation.
/*
* Copyright 2006-2012, Rathravane LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.rathravane.drumlin.service.framework.routing.playish;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.slf4j.LoggerFactory;
import com.rathravane.drumlin.service.framework.DrumlinConnection;
import com.rathravane.drumlin.service.framework.context.DrumlinRequestContext;
import com.rathravane.drumlin.service.framework.routing.DrumlinRouteInvocation;
import com.rathravane.drumlin.service.framework.routing.DrumlinRouteSource;
/**
* A static entry point routing source is a collection of routing entries for mapping request
* paths to static files and directories.
*
* @author [email protected]
*
*/
public class DrumlinPlayishStaticEntryPointRoutingSource implements DrumlinRouteSource
{
public DrumlinPlayishStaticEntryPointRoutingSource ()
{
fPathList = new LinkedList<>();
fPackages = new LinkedList<>();
}
/**
* Add a verb and path route with an action string. The action can start with "staticDir:" or
* "staticFile:". The remainder of the string is used as a relative filename to the dir (staticDir:), or
* as a filename (staticFile:).
* @param verb
* @param path
* @param action
* @return this object (for use in chaining the add calls)
*/
public synchronized DrumlinPlayishStaticEntryPointRoutingSource addRoute ( String verb, String path, String action )
{
if ( action.startsWith ( kStaticDirTag ) )
{
final DrumlinPathInfo pe = DrumlinPathInfo.processPath ( verb, path + ".*" );
pe.setHandler ( new StaticDirHandler ( path, action.substring ( kStaticDirTag.length () ) ) );
fPathList.add ( pe );
}
else if ( action.startsWith ( kStaticFileTag ) )
{
final DrumlinPathInfo pe = DrumlinPathInfo.processPath ( verb, path );
pe.setHandler ( new StaticFileHandler ( path, action.substring ( kStaticFileTag.length () ) ) );
fPathList.add ( pe );
}
else if ( action.startsWith ( kRedirectTag ) )
{
final DrumlinPathInfo pe = DrumlinPathInfo.processPath ( verb, path );
final String loc = action.substring ( kRedirectTag.length () );
pe.setHandler ( new RedirectHandler ( loc ) );
fPathList.add ( pe );
}
else if ( action.startsWith ( kTemplateDirTag ) )
{
final DrumlinPathInfo pe = DrumlinPathInfo.processPath ( verb, path + ".*" );
pe.setHandler ( new TemplateDirHandler ( "/" + action.substring ( kTemplateDirTag.length () ) ) );
fPathList.add ( pe );
}
else
{
final DrumlinPathInfo pe = DrumlinPathInfo.processPath ( verb, path );
pe.setHandler ( new StaticJavaEntryAction ( action, pe.getArgs(), fPackages ) );
fPathList.add ( pe );
}
return this;
}
/**
* Get a route invocation for a given verb+path, or null.
*/
@Override
public synchronized DrumlinRouteInvocation getRouteFor ( String verb, String path, DrumlinConnection forSession )
{
DrumlinRouteInvocation selected = null;
for ( DrumlinPathInfo pe : fPathList )
{
final List args = pe.matches ( verb, path );
if ( args != null )
{
selected = getInvocation ( pe, args );
break;
}
}
return selected;
}
/**
* Get the URL that reaches a given static method with the given arguments.
*/
@Override
public String getRouteTo ( Class> c, String staticMethodName, Map args, DrumlinConnection forSession )
{
final String fullname = c.getName() + "." + staticMethodName;
for ( DrumlinPathInfo pe : fPathList )
{
if ( pe.invokes ( fullname ) )
{
return pe.makePath ( args );
}
}
return null;
}
private final LinkedList fPackages;
private final LinkedList fPathList;
private static final org.slf4j.Logger log = LoggerFactory.getLogger ( DrumlinPlayishStaticEntryPointRoutingSource.class );
protected invocation getInvocation ( DrumlinPathInfo pe, List args )
{
return new invocation ( pe, args );
}
protected class invocation implements DrumlinRouteInvocation
{
public invocation ( DrumlinPathInfo pe, List args )
{
fPe = pe;
fArgs = args;
}
@Override
public void run ( DrumlinRequestContext ctx ) throws IOException, IllegalArgumentException, IllegalAccessException, InvocationTargetException
{
fPe.getHandler ().handle ( ctx, fArgs );
}
private final DrumlinPathInfo fPe;
private final List fArgs;
}
protected synchronized void clearRoutes ()
{
log.debug ( "Clearing routes within this static route source." );
fPathList.clear ();
}
protected synchronized void addPackage ( String pkg )
{
fPackages.add ( pkg );
}
private static final String kStaticDirTag = "staticDir:";
private static final String kStaticFileTag = "staticFile:";
private static final String kRedirectTag = "redirect:";
private static final String kTemplateDirTag = "templateDir:";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy