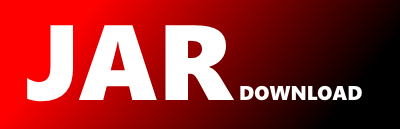
com.rathravane.till.console.shell.simpleCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of silt Show documentation
Show all versions of silt Show documentation
A small collection of classes used in various Rathravane systems.
/*
* Copyright 2006-2012, Rathravane LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.rathravane.till.console.shell;
import java.io.PrintStream;
import java.util.HashMap;
import com.rathravane.till.console.CmdLineParser;
import com.rathravane.till.console.CmdLinePrefs;
import com.rathravane.till.console.ConsoleProgram.usageException;
import com.rathravane.till.nv.rrNvReadable;
public abstract class simpleCommand implements command
{
protected simpleCommand ( String cmd )
{
this ( cmd, cmd, null );
}
protected simpleCommand ( String cmd, String usage )
{
this ( cmd, usage, null );
}
protected simpleCommand ( String cmd, String usage, String help )
{
fCmd = cmd;
fUsage = usage;
fHelp = help;
fArgsParser = new CmdLineParser ();
fPrefs = null;
fEnabled = true;
}
public void enable ( boolean e )
{
fEnabled = e;
}
public boolean enabled ()
{
return fEnabled;
}
@Override
public final void checkArgs ( rrNvReadable basePrefs, String[] args ) throws usageException
{
setupParser ( fArgsParser );
fPrefs = fArgsParser.processArgs ( args );
}
@Override
public String getCommand () { return fCmd; }
@Override
public String getUsage () { return fUsage; }
@Override
public String getHelp () { return fHelp; }
@Override
public final consoleLooper.inResult execute ( HashMap workspace, PrintStream outTo ) throws usageException
{
try
{
return execute ( workspace, fPrefs, outTo );
}
catch ( rrNvReadable.missingReqdSetting e )
{
throw new usageException ( e );
}
}
/**
* Override this to run the command.
* @param workspace
* @param p
* @param outTo
* @return true to continue, false to exit
* @throws usageException
* @throws rrNvReadable.missingReqdSetting
*/
protected abstract consoleLooper.inResult execute ( HashMap workspace, CmdLinePrefs p, PrintStream outTo ) throws usageException, rrNvReadable.missingReqdSetting;
/**
* override this to specify arguments for the command
* @param clp
*/
protected void setupParser ( CmdLineParser clp ) {}
private final String fCmd;
private final String fUsage;
private final String fHelp;
private final CmdLineParser fArgsParser;
private CmdLinePrefs fPrefs;
private boolean fEnabled;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy