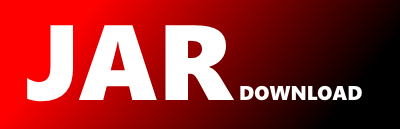
com.rathravane.till.data.json.JsonUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of silt Show documentation
Show all versions of silt Show documentation
A small collection of classes used in various Rathravane systems.
package com.rathravane.till.data.json;
import java.io.InputStream;
import java.io.Reader;
import java.util.Collections;
import java.util.Comparator;
import java.util.LinkedList;
import java.util.List;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.rathravane.till.data.json.JsonVisitor.ArrayOfObjectVisitor;
import com.rathravane.till.data.json.JsonVisitor.ArrayOfStringVisitor;
import com.rathravane.till.data.json.JsonVisitor.ObjectVisitor;
public class JsonUtil
{
public static JSONObject readJsonObject ( InputStream is )
{
return new JSONObject ( new CommentedJsonTokener ( is ) );
}
public static JSONObject readJsonObject ( String is )
{
return new JSONObject ( new CommentedJsonTokener ( is ) );
}
public static JSONObject readJsonObject ( Reader is )
{
return new JSONObject ( new CommentedJsonTokener ( is ) );
}
public static JSONArray readJsonArray ( InputStream is )
{
return new JSONArray ( new CommentedJsonTokener ( is ) );
}
public static JSONArray readJsonArray ( String is )
{
return new JSONArray ( new CommentedJsonTokener ( is ) );
}
public static JSONArray readJsonArray ( Reader is )
{
return new JSONArray ( new CommentedJsonTokener ( is ) );
}
public static JSONObject clone ( JSONObject that )
{
if ( that == null ) return null;
final JSONObject result = new JSONObject ();
JsonVisitor.forEachElement ( that, new ObjectVisitor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy