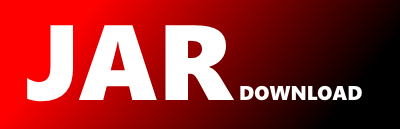
com.rathravane.till.data.json.JsonVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of silt Show documentation
Show all versions of silt Show documentation
A small collection of classes used in various Rathravane systems.
package com.rathravane.till.data.json;
import java.util.Collection;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
public class JsonVisitor
{
public interface ArrayVisitor
{
/**
* Visit an array entry. Return false to stop iteration.
* @param t
* @return
* @throws JSONException
* @throws E
*/
boolean visit ( T t ) throws JSONException, E;
}
public interface ArrayOfObjectVisitor extends ArrayVisitor
{
}
public interface ArrayOfStringVisitor extends ArrayVisitor
{
}
@SuppressWarnings("unchecked")
public static void forEachElement ( JSONArray a, ArrayVisitor v ) throws JSONException, E
{
if ( a == null ) return;
final int len = a.length ();
for ( int i=0; i objectToMap ( JSONObject obj )
{
final HashMap map = new HashMap ();
if ( obj != null )
{
for ( Object oo : obj.keySet () )
{
final String key = oo.toString ();
final String val = obj.getString ( key );
map.put ( key, val );
}
}
return map;
}
public static boolean listContains ( JSONArray a, String t )
{
if ( a == null ) return false;
final int len = a.length ();
for ( int i=0; i arrayToList ( JSONArray a )
{
final LinkedList list = new LinkedList ();
if ( a != null )
{
forEachElement ( a, new ArrayVisitor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy