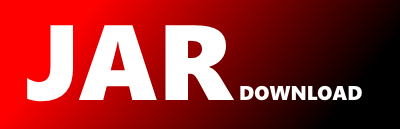
com.rathravane.till.data.xml.rrXmlReadHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of silt Show documentation
Show all versions of silt Show documentation
A small collection of classes used in various Rathravane systems.
package com.rathravane.till.data.xml;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.util.Collection;
import java.util.LinkedList;
import java.util.Map.Entry;
import java.util.logging.Logger;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.EntityResolver;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
/**
* Utilities for reading XML streams.
* @author peter
*
*/
public class rrXmlReadHelper
{
public static final String kTextContent = "_textContent";
public static Element getTopLevelElement ( byte[] docBytes )
{
final InputStream is = new ByteArrayInputStream ( docBytes );
return getTopLevelElement ( is );
}
public static Element getTopLevelElement ( InputStream is )
{
Element result = null;
try
{
final DocumentBuilderFactory f = DocumentBuilderFactory.newInstance ();
f.setValidating ( false );
f.setNamespaceAware ( false );
final DocumentBuilder db = f.newDocumentBuilder ();
db.setEntityResolver ( new EntityResolver ()
{
@Override
public InputSource resolveEntity ( String publicId, String systemId )
{
// it might be a good idea to insert a trace logging here
// that you are ignoring publicId/systemId
return new InputSource ( new StringReader ( "" ) );
}
} );
final Document d = db.parse ( is );
result = d.getDocumentElement ();
}
catch ( ParserConfigurationException e )
{
log.warning ( "XML parse error: " + e.getMessage () );
}
catch ( SAXException e )
{
log.warning ( "XML parse error: " + e.getMessage () );
}
catch ( IOException e )
{
log.warning ( "XML parse error: " + e.getMessage () );
}
return result;
}
public static Element getFirstNamedChildElement ( Element parent, String name )
{
Element result = null;
Collection elements = getNamedChildElements ( parent, name );
if ( elements.size () > 0 )
{
result = elements.iterator ().next ();
}
return result;
}
public static Node getFirstNamedChildNode ( Node parent, String name )
{
Node result = null;
Collection elements = getNamedChildNodes ( parent, name );
if ( elements.size () > 0 )
{
result = elements.iterator ().next ();
}
return result;
}
public static Collection getNamedChildElements ( Element parent, String name )
{
final LinkedList result = new LinkedList ();
final NodeList elements = parent.getElementsByTagName ( name );
for ( int i=0; i getNamedChildNodes ( Node parent, String name )
{
final LinkedList result = new LinkedList ();
final NodeList nodes = parent.getChildNodes ();
for ( int i=0; i getAllChildElements ( Node parent )
{
LinkedList result = new LinkedList ();
NodeList elements = parent.getChildNodes ();
for ( int i=0; i
{
public attrEntry ( String k, String v )
{
fKey = k;
fValue = v;
}
public String getKey () { return fKey; }
public String getValue () { return fValue; }
public String setValue ( String arg0 ) throws UnsupportedOperationException { throw new UnsupportedOperationException(); }
private final String fKey;
private final String fValue;
}
public static rrXmlAttrMap getAttrMap ( Node n )
{
return getAttrMap ( n, null );
}
public static rrXmlAttrMap getAttrMap ( Node n, rrXmlAttrMap inherited )
{
return getAttrMap ( n, inherited, true );
}
public static rrXmlAttrMap getAttrMap ( Node n, rrXmlAttrMap inherited, boolean withTextContent )
{
final rrXmlAttrMap result = new rrXmlAttrMap ( inherited );
for ( Entry e : getAllAttrs ( n, withTextContent ) )
{
result.put ( e.getKey (), e.getValue () );
}
return result;
}
public static Collection> getAllAttrs ( Node n, boolean withTextContent )
{
LinkedList> result = new LinkedList> ();
NamedNodeMap nnm = n.getAttributes ();
if ( nnm != null )
{
final int size = nnm.getLength ();
for ( int i=0; i { void onAttr ( Node parent, String name, String val ) throws T; };
public static void forEachAttr ( Node n, final attrAction aa ) throws T
{
for ( Entry e : getAllAttrs ( n, false ) )
{
aa.onAttr ( n, e.getKey(), e.getValue () );
}
}
public interface elementAction { void onElement ( Node parent, Element e ) throws T; };
public static void forEachElement ( Node n, final elementAction aa ) throws T
{
for ( Element e : getAllChildElements ( n ) )
{
aa.onElement ( n, e );
}
}
public static void forEachElementNamed ( Node n, String name, final elementAction aa ) throws T
{
for ( Element e : getAllChildElements ( n ) )
{
final String nodeName = e.getNodeName ();
if ( nodeName.equals ( name ) )
{
aa.onElement ( n, e );
}
}
}
private static final Logger log = Logger.getLogger ( rrXmlReadHelper.class.getName () );
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy