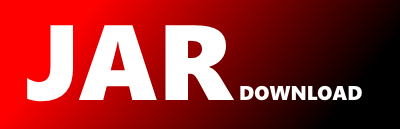
com.rathravane.till.persistence.ResourceLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of silt Show documentation
Show all versions of silt Show documentation
A small collection of classes used in various Rathravane systems.
package com.rathravane.till.persistence;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.services.s3.AmazonS3Client;
import com.amazonaws.services.s3.AmazonS3URI;
import com.amazonaws.services.s3.model.AmazonS3Exception;
import com.amazonaws.services.s3.model.GetObjectRequest;
import com.amazonaws.services.s3.model.S3Object;
import com.rathravane.till.data.rrStreamTools;
/**
* ResourceLoader is a general purpose tool for loading a data stream by name.
* @author peter
*
*/
public class ResourceLoader
{
public static InputStream load ( String resource ) throws IOException
{
return new ResourceLoader().named ( resource ).load ();
}
public ResourceLoader ()
{
}
public ResourceLoader named ( String name )
{
fNamed = name;
return this;
}
public InputStream load () throws IOException
{
if ( fNamed == null ) return null;
if ( fNamed.startsWith ( "s3://" ) )
{
return loadFromS3 ( fNamed );
}
else if ( fNamed.startsWith ( "http://" ) || fNamed.startsWith ( "https://" ) )
{
return loadHttp ( fNamed );
}
// otherwise...
InputStream is = null;
// try as a file
final File f = new File ( fNamed );
if ( f.exists () )
{
try
{
return new FileInputStream ( fNamed );
}
catch ( FileNotFoundException x )
{
log.warn ( "File not found after exists() returned true.", x );
}
}
is = ResourceLoader.class.getClassLoader().getResourceAsStream ( fNamed );
if ( is != null )
{
return is;
}
// now try the system class loaders' resource finder
is = ClassLoader.getSystemResourceAsStream ( fNamed );
if ( is != null )
{
return is;
}
return null;
}
private String fNamed = null;
private static final Logger log = LoggerFactory.getLogger ( ResourceLoader.class );
private static InputStream loadFromS3 ( String url ) throws IOException
{
final AmazonS3URI uri = new AmazonS3URI ( url );
final AWSCredentials creds = new AWSCredentials ()
{
@Override
public String getAWSAccessKeyId () { return System.getenv ( "AWS_ACCESS_KEY_ID" ); }
@Override
public String getAWSSecretKey () { return System.getenv ( "AWS_SECRET_ACCESS_KEY" ); }
};
final AmazonS3Client db = new AmazonS3Client ( creds );
final ByteArrayOutputStream baos = new ByteArrayOutputStream ();
S3Object object = null;
try
{
object = db.getObject ( new GetObjectRequest ( uri.getBucket (), uri.getKey () ) );
final InputStream is = object.getObjectContent ();
// s3 objects must be closed or will leak an HTTP connection
rrStreamTools.copyStream ( is, baos );
}
catch ( AmazonS3Exception x )
{
throw new IOException ( x );
}
finally
{
if ( object != null )
{
object.close ();
}
}
return new ByteArrayInputStream ( baos.toByteArray () );
}
private static InputStream loadHttp ( String url ) throws IOException
{
return new URL ( url ).openStream ();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy