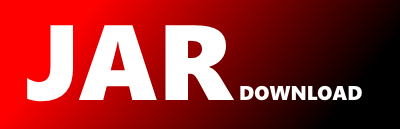
com.rationaleemotions.ScpDownloadFileWorker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simple-ssh Show documentation
Show all versions of simple-ssh Show documentation
A simple way of interacting with a remote host for executing commands, scp (upload and download)
package com.rationaleemotions;
import com.jcraft.jsch.ChannelSftp;
import com.jcraft.jsch.JSchException;
import com.jcraft.jsch.Session;
import com.jcraft.jsch.SftpException;
import com.rationaleemotions.pojo.ExecResults;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.util.LinkedList;
import java.util.List;
import java.util.concurrent.Callable;
/**
* A Worker that is capable of downloading a file from a remote host.
*/
class ScpDownloadFileWorker implements Callable {
interface Marker {}
private static final Logger LOGGER = LoggerFactory.getLogger(Marker.class.getEnclosingClass());
private ChannelSftp channel;
private File file;
private String remoteLoc;
ScpDownloadFileWorker(Session session, File file, String remoteLoc) throws JSchException {
channel = (ChannelSftp) session.openChannel("sftp");
this.file = file;
this.remoteLoc = remoteLoc;
}
@Override
public ExecResults call() throws Exception {
int rc;
List error = new LinkedList<>();
try {
channel.connect();
channel.get(remoteLoc, file.getAbsolutePath());
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Completed downloading [" + file.getAbsolutePath() + "] to [" + remoteLoc + "]");
}
} catch (JSchException | SftpException e) {
String msg = "Encountered problems when downloading [" + remoteLoc + "]. Root cause : " + e.getMessage();
error.add(msg);
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("Failed downloading [" + file.getAbsolutePath() + "] to [" + remoteLoc + "]", e);
}
} finally {
rc = channel.getExitStatus();
channel.disconnect();
}
return new ExecResults(new LinkedList<>(), error, rc);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy