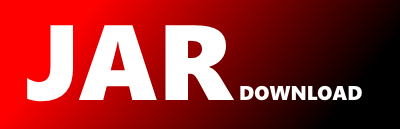
com.rationaleemotions.utils.StreamGuzzler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simple-ssh Show documentation
Show all versions of simple-ssh Show documentation
A simple way of interacting with a remote host for executing commands, scp (upload and download)
package com.rationaleemotions.utils;
import com.rationaleemotions.ExecutionFailedException;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.LinkedList;
import java.util.List;
/**
* A simple thread based utility that consumes {@link InputStream} and provides the stream contents
* as a list of strings.
* This utility is NOT designed to be re-used. So everytime a stream is to be consumed, a new
* object of this utility is expected to be created. Attempting to re-use an existing instance of {@link StreamGuzzler}
* for consuming stream will trigger errors.
*/
public class StreamGuzzler implements Runnable {
private InputStream stream;
private List content = new LinkedList<>();
public List getContent() {
return content;
}
public StreamGuzzler(InputStream stream) {
this.stream = stream;
}
@Override
public void run() {
Preconditions.checkState(stream != null, "Cannot guzzle an empty/null stream.");
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(stream));
String line;
while ((line = reader.readLine()) != null) {
content.add(line);
}
} catch (IOException exception) {
throw new ExecutionFailedException(exception);
} finally {
this.stream = null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy