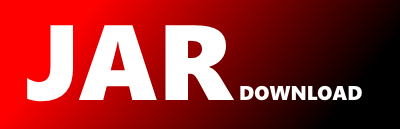
com.recombee.api_client.api_requests.Batch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api-client Show documentation
Show all versions of api-client Show documentation
A client library for easy use of the Recombee recommendation API
package com.recombee.api_client.api_requests;
import java.util.Map;
import java.util.HashMap;
import java.util.List;
import java.util.ArrayList;
import java.util.Arrays;
import com.recombee.api_client.util.HTTPMethod;
/**
* In many cases, it may be desirable to execute multiple requests at once. For example, when synchronizing the catalog of items in periodical manner, you would have to execute a sequence of thousands of separate POST requests, which is very ineffective and may take a very long time to complete. Most notably, network latencies can make execution of such a sequence very slow and even if executed in multiple parallel threads, there will still be unreasonable overhead caused by the HTTP(s). To avoid the problems mentioned, batch processing may be used, encapsulating a sequence of requests into a single HTTP request.
* Batch processing allows you to submit arbitrary sequence of requests in form of JSON array. Any type of request from the above documentation may be used in the batch, and the batch may combine different types of requests arbitrarily as well.
* Note that:
* - executing the requests in a batch is equivalent as if they were executed one-by-one individually; there are, however, many optimizations to make batch execution as fast as possible,
* - the status code of the batch request itself is 200 even if the individual requests result in error – you have to inspect the code values in the resulting array,
* - if the status code of the whole batch is not 200, then there is an error in the batch request itself; in such a case, the error message returned should help you to resolve the problem,
* - currently, batch size is limited to **10,000** requests; if you wish to execute even larger number of requests, please split the batch into multiple parts.
*/
public class Batch extends Request {
/**
* Requests contained in the batch
*/
protected List requests;
protected boolean distinctRecomms;
/**
* Construct the request
* @param requests Requests which should be processed in a single batch request
*/
public Batch (List requests) {
initialize(requests);
}
/**
* Construct the request
* @param requests Requests which should be processed in a single batch request
*/
public Batch(Request[] requests)
{
initialize(Arrays.asList(requests));
}
private void initialize(List requests)
{
this.requests = requests;
this.distinctRecomms = false;
this.timeout = 0;
for(Request r: this.requests)
this.timeout += r.getTimeout();
}
public List getRequests() {
return this.requests;
}
/**
* @param distinctRecomms Makes all the recommended items for a certain user distinct among multiple recommendation requests in the batch
*/
public Batch setDistinctRecomms(boolean distinctRecomms) {
this.distinctRecomms = distinctRecomms;
return this;
}
public boolean getDistinctRecomms() {
return this.distinctRecomms;
}
/**
* @return Used HTTP method
*/
@Override
public HTTPMethod getHTTPMethod() {
return HTTPMethod.POST;
}
/**
* Returns true if HTTPS must be chosen over HTTP for this request
* @return True if HTTPS must be chosen
*/
@Override
public boolean getEnsureHttps() {
return true;
}
/**
* @return URI to the endpoint
*/
@Override
public String getPath() {
return "/batch/";
}
/**
* Get query parameters
* @return Values of query parameters (name of parameter: value of the parameter)
*/
@Override
public Map getQueryParameters() {
return new HashMap();
}
/**
* Get body parameters
* @return Values of body parameters (name of parameter: value of the parameter)
*/
@Override
public Map getBodyParameters() {
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy