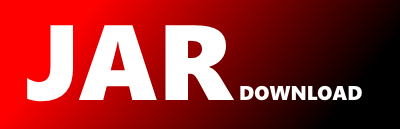
com.recombee.api_client.api_requests.AddRating Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api-client Show documentation
Show all versions of api-client Show documentation
A client library for easy use of the Recombee recommendation API
package com.recombee.api_client.api_requests;
/*
This file is auto-generated, do not edit
*/
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.recombee.api_client.bindings.Logic;
import com.recombee.api_client.util.HTTPMethod;
/**
* Adds a rating of given item made by a given user.
*/
public class AddRating extends Request {
/**
* User who submitted the rating
*/
protected String userId;
/**
* Rated item
*/
protected String itemId;
/**
* UTC timestamp of the rating as ISO8601-1 pattern or UTC epoch time. The default value is the current time.
*/
protected Date timestamp;
/**
* Rating rescaled to interval [-1.0,1.0], where -1.0 means the worst rating possible, 0.0 means neutral, and 1.0 means absolutely positive rating. For example, in the case of 5-star evaluations, rating = (numStars-3)/2 formula may be used for the conversion.
*/
protected Double rating;
/**
* Sets whether the given user/item should be created if not present in the database.
*/
protected Boolean cascadeCreate;
/**
* If this rating is based on a recommendation request, `recommId` is the id of the clicked recommendation.
*/
protected String recommId;
/**
* A dictionary of additional data for the interaction.
*/
protected Map additionalData;
/**
* Construct the request
* @param userId User who submitted the rating
* @param itemId Rated item
* @param rating Rating rescaled to interval [-1.0,1.0], where -1.0 means the worst rating possible, 0.0 means neutral, and 1.0 means absolutely positive rating. For example, in the case of 5-star evaluations, rating = (numStars-3)/2 formula may be used for the conversion.
*/
public AddRating (String userId,String itemId,double rating) {
this.userId = userId;
this.itemId = itemId;
this.rating = rating;
this.timeout = 1000;
}
/**
* @param timestamp UTC timestamp of the rating as ISO8601-1 pattern or UTC epoch time. The default value is the current time.
*/
public AddRating setTimestamp(Date timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* @param cascadeCreate Sets whether the given user/item should be created if not present in the database.
*/
public AddRating setCascadeCreate(boolean cascadeCreate) {
this.cascadeCreate = cascadeCreate;
return this;
}
/**
* @param recommId If this rating is based on a recommendation request, `recommId` is the id of the clicked recommendation.
*/
public AddRating setRecommId(String recommId) {
this.recommId = recommId;
return this;
}
/**
* @param additionalData A dictionary of additional data for the interaction.
*/
public AddRating setAdditionalData(Map additionalData) {
this.additionalData = additionalData;
return this;
}
public String getUserId() {
return this.userId;
}
public String getItemId() {
return this.itemId;
}
public Date getTimestamp() {
return this.timestamp;
}
public double getRating() {
return this.rating;
}
public boolean getCascadeCreate() {
if (this.cascadeCreate==null) return false;
return this.cascadeCreate;
}
public String getRecommId() {
return this.recommId;
}
public Map getAdditionalData() {
return this.additionalData;
}
/**
* @return Used HTTP method
*/
@Override
public HTTPMethod getHTTPMethod() {
return HTTPMethod.POST;
}
/**
* @return URI to the endpoint including path parameters
*/
@Override
public String getPath() {
return "/ratings/";
}
/**
* Get query parameters
* @return Values of query parameters (name of parameter: value of the parameter)
*/
@Override
public Map getQueryParameters() {
HashMap params = new HashMap();
return params;
}
/**
* Get body parameters
* @return Values of body parameters (name of parameter: value of the parameter)
*/
@Override
public Map getBodyParameters() {
HashMap params = new HashMap();
params.put("userId", this.userId);
params.put("itemId", this.itemId);
params.put("rating", this.rating);
if (this.timestamp!=null) {
params.put("timestamp", this.timestamp.getTime()/1000.0);
}
if (this.cascadeCreate!=null) {
params.put("cascadeCreate", this.cascadeCreate);
}
if (this.recommId!=null) {
params.put("recommId", this.recommId);
}
if (this.additionalData!=null) {
params.put("additionalData", this.additionalData);
}
return params;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy