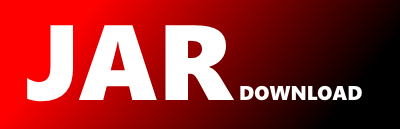
com.redhat.darcy.ui.api.ElementSelection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of darcy-ui Show documentation
Show all versions of darcy-ui Show documentation
Framework for writing page objects to automate interaction with graphical user interfaces.
The newest version!
/*
Copyright 2014 Red Hat, Inc. and/or its affiliates.
This file is part of darcy-ui.
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see .
*/
package com.redhat.darcy.ui.api;
import com.redhat.darcy.ui.api.elements.Button;
import com.redhat.darcy.ui.api.elements.Checkbox;
import com.redhat.darcy.ui.api.elements.DateInput;
import com.redhat.darcy.ui.api.elements.Element;
import com.redhat.darcy.ui.api.elements.FileSelect;
import com.redhat.darcy.ui.api.elements.Label;
import com.redhat.darcy.ui.api.elements.Link;
import com.redhat.darcy.ui.api.elements.MultiSelect;
import com.redhat.darcy.ui.api.elements.Radio;
import com.redhat.darcy.ui.api.elements.Select;
import com.redhat.darcy.ui.api.elements.Text;
import com.redhat.darcy.ui.api.elements.TextInput;
import com.redhat.darcy.ui.internal.ChainedViewFactory;
import com.redhat.darcy.ui.internal.NestedViewFactory;
import java.util.List;
/**
* A {@link Selection} for {@link com.redhat.darcy.ui.api.elements.Element}s.
*
* @see ElementContext
*/
public interface ElementSelection extends Selection {
/**
* Retrieve a reference to a single element of some type. The returned element reference may or
* may not be found within the context. This is to say that if an element is not found
* by the given locator, no exception will be thrown, and an element of that type will still be
* returned. An exception will be thrown, however, if you try to act on that element in a way
* that requires its presence. You can safely determine whether an element was found by calling
* {@link com.redhat.darcy.ui.api.elements.Element#isDisplayed()}.
*
* @param elementType The type of element reference to retrieve. Whether or not the automation
* library enforces the found element actually be of the desired type is implementation
* specific.
* @param locator
* @return
*/
T elementOfType(Class elementType, Locator locator);
/**
* Retrieve a list of elements. Only found elements will be contained within the list. List may
* only reflect elements found at the moment a method on the list is called, or it may reflect
* elements found at the time of retrieving the list.
*
* @param elementType The type of element reference to retrieve. Whether or not the automation
* library enforces the found element actually be of the desired type is implementation
* specific.
* @param locator
* @return
*/
List elementsOfType(Class elementType, Locator locator);
T viewOfType(ChainedViewFactory elementCtor, Locator locator);
List viewsOfType(NestedViewFactory elementCtor, Locator locator);
default Element element(Locator locator) {
return elementOfType(Element.class, locator);
}
default List elements(Locator locator) {
return elementsOfType(Element.class, locator);
}
default TextInput textInput(Locator locator) {
return elementOfType(TextInput.class, locator);
}
default List textInputs(Locator locator) {
return elementsOfType(TextInput.class, locator);
}
default Button button(Locator locator) {
return elementOfType(Button.class, locator);
}
default List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy