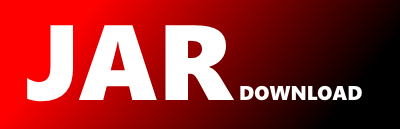
com.redhat.insights.reports.UpdateReportImpl Maven / Gradle / Ivy
/* Copyright (C) Red Hat 2023-2024 */
package com.redhat.insights.reports;
import com.fasterxml.jackson.databind.JsonSerializer;
import com.redhat.insights.Filtering;
import com.redhat.insights.jars.JarInfo;
import com.redhat.insights.jars.JarInfoSubreport;
import com.redhat.insights.logging.InsightsLogger;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.BlockingQueue;
/** An {@code UPDATE} event report implementation. */
public class UpdateReportImpl implements InsightsReport {
private String idHash = "";
private final BlockingQueue updatedJars;
private final InsightsLogger logger;
private Optional subreport = Optional.empty();
private final InsightsReportSerializer serializer;
public UpdateReportImpl(BlockingQueue updatedJars, InsightsLogger logger) {
this.updatedJars = updatedJars;
this.logger = logger;
this.serializer = new InsightsReportSerializer();
}
@Override
public Map getSubreports() {
if (!subreport.isPresent()) {
return Collections.emptyMap();
}
return Collections.singletonMap("updated-jars", subreport.get());
}
@Override
public JsonSerializer getSerializer() {
return serializer;
}
@Override
public void generateReport(Filtering __) {
if (!updatedJars.isEmpty()) {
List jars = new ArrayList<>();
int sendCount = updatedJars.drainTo(jars);
JarInfoSubreport jarInfoSubreport = new JarInfoSubreport(logger, jars);
jarInfoSubreport.generateReport();
subreport = Optional.of(jarInfoSubreport);
logger.debug("Sending " + sendCount + " jars from " + getIdHash());
}
}
@Override
public Map getBasic() {
return Collections.emptyMap();
}
@Override
public String getVersion() {
return "1.0.0";
}
@Override
public void setIdHash(String idHash) {
this.idHash = idHash;
}
@Override
public String getIdHash() {
return idHash;
}
@Override
public void decorate(String key, String value) {
logger.debug(
String.format("Attempt to add %s => %s to an update report. Ignored.", key, value));
}
@Override
public void close() throws IOException {}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy