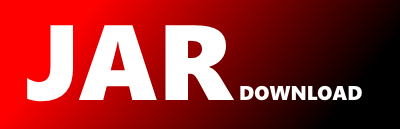
com.redhat.lightblue.crud.CRUDOperationContext Maven / Gradle / Ivy
/*
Copyright 2013 Red Hat, Inc. and/or its affiliates.
This file is part of lightblue.
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see .
*/
package com.redhat.lightblue.crud;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.HashSet;
import java.util.Iterator;
import com.redhat.lightblue.DataError;
import com.redhat.lightblue.ExecutionOptions;
import com.redhat.lightblue.ResultMetadata;
import com.redhat.lightblue.hooks.HookManager;
import com.redhat.lightblue.util.Error;
import com.redhat.lightblue.util.JsonDoc;
import com.redhat.lightblue.util.Measure;
/**
* An implementation of this class is passed into CRUD operation
* implementations. It contains information about the caller roles, correct
* metadata versions, and the constraint validators that will be used in this
* call.
*/
public abstract class CRUDOperationContext implements MetadataResolver, Serializable {
private static final long serialVersionUID = 1l;
private final Factory factory;
private final String entityName;
private final Set callerRoles;
// We are going to use DocumentStream instead of list of documents for find results
private List documents;
private DocumentStream documentStream;
private final List errors = new ArrayList<>();
private final Map propertyMap = new HashMap<>();
private final CRUDOperation CRUDOperation;
private final HookManager hookManager;
private final ExecutionOptions executionOptions;
private final Set documentVersions=new HashSet<>();
private boolean updateIfCurrent;
private boolean computeCounts=true;
private boolean limitQueryTime=true;
public final Measure measure=new Measure();
/**
* This is the constructor used to represent the context of an operation
*/
public CRUDOperationContext(CRUDOperation op,
String entityName,
Factory f,
List docs,
ExecutionOptions eo) {
this.CRUDOperation = op;
this.entityName = entityName;
this.factory = f;
if(docs!=null) {
setInputDocuments(docs.stream().map(x->new DocCtx(x)).collect(Collectors.toList()));
}
this.hookManager = new HookManager(factory.getHookResolver(), factory.getNodeFactory());
this.callerRoles = new HashSet<>();
this.executionOptions = eo;
}
public CRUDOperationContext(CRUDOperation op,
String entityName,
Factory f,
Set callerRoles,
HookManager hookManager,
List docs,
ExecutionOptions eo) {
this.CRUDOperation = op;
this.entityName = entityName;
this.factory = f;
if(docs!=null) {
setInputDocuments(docs.stream().map(x->new DocCtx(x)).collect(Collectors.toList()));
}
this.callerRoles = callerRoles;
this.hookManager = hookManager;
this.executionOptions = eo;
}
/**
* This constructor is used to construct an operation context that is
* derived from another existing context.
*/
public CRUDOperationContext(CRUDOperation op,
String entityName,
Factory f,
List docs,
Set callerRoles,
HookManager hookManager,
ExecutionOptions eo) {
this.CRUDOperation = op;
this.entityName = entityName;
this.factory = f;
setInputDocuments(docs);
this.callerRoles = callerRoles;
this.hookManager = hookManager;
this.executionOptions = eo;
}
/**
* If this list is non-empty, then update operations should be
* performed only if document versions are unchanged
*/
public Set getUpdateDocumentVersions() {
return documentVersions;
}
/**
* If true, then only update the documents in
* updateDocumentVersions set, and update them only if they are
* unchanged
*/
public boolean isUpdateIfCurrent() {
return updateIfCurrent;
}
public void setUpdateIfCurrent(boolean b) {
updateIfCurrent=b;
}
public boolean isLimitQueryTime() {
return limitQueryTime;
}
public void setLimitQueryTime(boolean b) {
limitQueryTime=b;
}
/**
* Returns the execution options
*/
public ExecutionOptions getExecutionOptions() {
return executionOptions;
}
/**
* Returns the entity name in the context
*/
public String getEntityName() {
return entityName;
}
/**
* Returns the factory instance that controls the validator and CRUD
* instances.
*/
public Factory getFactory() {
return factory;
}
public boolean isComputeCounts() {
return computeCounts;
}
public void setComputeCounts(boolean b) {
computeCounts=b;
}
/**
* Sets the caller roles
*/
protected void addCallerRoles(Set roles) {
this.callerRoles.addAll(roles);
}
/**
* Returns the roles the caller is in
*/
public Set getCallerRoles() {
return callerRoles;
}
public void setDocumentStream(DocumentStream stream) {
this.documentStream=stream;
}
/**
* Returns the documents as a stream. This will be used for result retrieval
* only. If the back-end sets the documet stream, then it will be used.
* If the back-end sets the document list, a ListDocumentStream will be returned
* to iterate through those documents.
*/
public DocumentStream getDocumentStream() {
return documentStream;
}
/**
* Returns the list of input documents in the context.
*/
public List getInputDocuments() {
return documents;
}
public void setInputDocuments(List docs) {
documents = docs;
if(documents!=null) {
// Set the documentStream to input documents. A later call to setDocumentStream may set the output stream
documentStream=new ListDocumentStream(documents);
}
}
/**
* Returns the current operation
*/
public CRUDOperation getCRUDOperation() {
return CRUDOperation;
}
/**
* Returns a list of documents with no errors
*/
public List getInputDocumentsWithoutErrors() {
if (documents != null) {
List list = new ArrayList<>(documents.size());
for (DocCtx doc : documents) {
if (!doc.hasErrors()) {
list.add(doc);
}
}
return list;
} else {
return null;
}
}
/**
* Returns if there are documents with no errors
*/
public boolean hasInputDocumentsWithoutErrors() {
if (documents != null) {
for (DocCtx x : documents) {
if (!x.hasErrors()) {
return true;
}
}
}
return false;
}
/**
* Adds an error to the context
*/
public void addError(Error e) {
errors.add(e);
}
/**
* Adds errors to the context
*/
public void addErrors(Collection l) {
errors.addAll(l);
}
/**
* Returns the list of errors
*/
public List getErrors() {
return errors;
}
/**
* Returns if there are any errors. This does not take into account document
* errors.
*/
public boolean hasErrors() {
return !errors.isEmpty();
}
/**
* Properties for the context
*/
public Object getProperty(String name) {
return propertyMap.get(name);
}
/**
* Properties for the context
*/
public void setProperty(String name, Object value) {
propertyMap.put(name, value);
}
/**
* The hookManager for this operation
*/
public HookManager getHookManager() {
return hookManager;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy