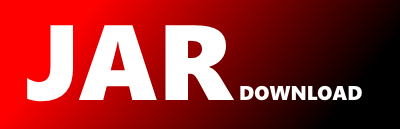
com.redhat.lightblue.metadata.parser.Extensions Maven / Gradle / Ivy
/*
Copyright 2013 Red Hat, Inc. and/or its affiliates.
This file is part of lightblue.
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see .
*/
package com.redhat.lightblue.metadata.parser;
import com.redhat.lightblue.metadata.DataStore;
import com.redhat.lightblue.metadata.EntityConstraint;
import com.redhat.lightblue.metadata.FieldConstraint;
import com.redhat.lightblue.metadata.HookConfiguration;
import com.redhat.lightblue.util.DefaultRegistry;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Parser extensions where T is the node type of the underlying object tree (for
* JSon, T is JsonNode).
*/
public class Extensions {
private static final Logger LOGGER = LoggerFactory.getLogger(Extensions.class);
private final ParserRegistry backendParsers = new ParserRegistry<>();
private final ParserRegistry entityConstraintParsers = new ParserRegistry<>();
private final ParserRegistry fieldConstraintParsers = new ParserRegistry<>();
private final ParserRegistry hookConfigurationParsers = new ParserRegistry<>();
private final DefaultRegistry> propertyParsers = new DefaultRegistry<>();
private final PropertyParser defaultPropertyParser = new PropertyParser<>();
/**
* Initializes this to include the default extensions
*/
public void addDefaultExtensions() {
fieldConstraintParsers.add(new DefaultFieldConstraintParsers());
entityConstraintParsers.add(new DefaultEntityConstraintParsers());
LOGGER.debug("Initialized addDefaultExtensions");
}
/**
* Adds a parser that parses DataStore
*
* @param name Name of the backend type (such as 'mongo')
* @param parser The parser that parses backend of the given type
*/
public void registerDataStoreParser(String name, DataStoreParser parser) {
backendParsers.add(name, parser);
}
/**
* Gets a backend parser
*
* @param backendName Name of the backend (such as 'mongo')
*
* @return The parser for the backend, or null if it does not exist
*/
public DataStoreParser getDataStoreParser(String backendName) {
return (DataStoreParser) backendParsers.find(backendName);
}
/**
* Registers an entity constraint parser for a given constraint name.
*
* @param name Name of the constraint
* @param parser The parser for the constraint
*/
public void registerEntityConstraintParser(String name, EntityConstraintParser parser) {
entityConstraintParsers.add(name, parser);
}
/**
* Returns an entity constraint parser
*
* @param constraintName
*
* @return The parser that parses the constraint, or null if a parser is not
* found
*/
public EntityConstraintParser getEntityConstraintParser(String constraintName) {
return (EntityConstraintParser) entityConstraintParsers.find(constraintName);
}
/**
* Adds a contraint parser for a given constraint name
*
* @param name Name of the constraint
* @param parser The parser for the constraint
*
*/
public void registerFieldConstraintParser(String name, FieldConstraintParser< T> parser) {
fieldConstraintParsers.add(name, parser);
}
/**
* Returns a parser that parses the given constraint
*
* @param constraintName Name of the constraint
*
* @return The parser that parses the constraint, or null if parser is not
* found
*/
public FieldConstraintParser getFieldConstraintParser(String constraintName) {
return (FieldConstraintParser) fieldConstraintParsers.find(constraintName);
}
/**
* Adds a hook configuration parser for a given hook name
*
* @param name Name of the hook
* @param parser The parser for the hook configuration
*
*/
public void registerHookConfigurationParser(String name, HookConfigurationParser parser) {
hookConfigurationParsers.add(name, parser);
}
/**
* Returns a parser that parses the given hook configuration
*
* @param hookName Name of the hook
*
* @return The parser that parses the hook configuration, or null if parser
* is not found
*/
public HookConfigurationParser getHookConfigurationParser(String hookName) {
return (HookConfigurationParser) hookConfigurationParsers.find(hookName);
}
public void registerPropertyParser(String propertyName, PropertyParser parser) {
propertyParsers.add(propertyName, parser);
}
public PropertyParser getPropertyParser(String propertyName) {
PropertyParser p = (PropertyParser) propertyParsers.find(propertyName);
return p == null ? defaultPropertyParser : p;
}
public void mergeWith(Extensions e) {
this.backendParsers.mergeWith(e.backendParsers);
this.entityConstraintParsers.mergeWith(e.entityConstraintParsers);
this.fieldConstraintParsers.mergeWith(e.fieldConstraintParsers);
this.hookConfigurationParsers.mergeWith(e.hookConfigurationParsers);
this.propertyParsers.mergeWith(e.propertyParsers);
}
@Override
public String toString() {
return backendParsers.toString() + "\n"
+ entityConstraintParsers.toString() + "\n"
+ fieldConstraintParsers.toString() + "\n"
+ hookConfigurationParsers.toString()
+ propertyParsers;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy