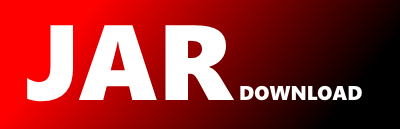
com.redis.lettucemod.api.async.RedisJSONAsyncCommands Maven / Gradle / Ivy
package com.redis.lettucemod.api.async;
import java.util.List;
import com.redis.lettucemod.json.ArrpopOptions;
import com.redis.lettucemod.json.GetOptions;
import com.redis.lettucemod.json.SetMode;
import com.redis.lettucemod.json.Slice;
import io.lettuce.core.KeyValue;
import io.lettuce.core.RedisFuture;
import io.lettuce.core.output.KeyValueStreamingChannel;
public interface RedisJSONAsyncCommands {
RedisFuture jsonDel(K key);
RedisFuture jsonDel(K key, String path);
@SuppressWarnings("unchecked")
RedisFuture jsonGet(K key, K... paths);
@SuppressWarnings("unchecked")
RedisFuture jsonGet(K key, GetOptions options, K... paths);
@SuppressWarnings("unchecked")
RedisFuture>> jsonMget(String path, K... keys);
@SuppressWarnings("unchecked")
RedisFuture jsonMget(KeyValueStreamingChannel channel, String path, K... keys);
RedisFuture jsonSet(K key, String path, V json);
RedisFuture jsonSet(K key, String path, V json, SetMode mode);
RedisFuture jsonMerge(K key, String path, V json);
RedisFuture jsonType(K key);
RedisFuture jsonType(K key, String path);
RedisFuture jsonNumincrby(K key, String path, double number);
RedisFuture jsonNummultby(K key, String path, double number);
RedisFuture jsonStrappend(K key, V json);
RedisFuture jsonStrappend(K key, String path, V json);
RedisFuture jsonStrlen(K key, String path);
@SuppressWarnings("unchecked")
RedisFuture jsonArrappend(K key, String path, V... jsons);
RedisFuture jsonArrindex(K key, String path, V scalar);
RedisFuture jsonArrindex(K key, String path, V scalar, Slice slice);
@SuppressWarnings("unchecked")
RedisFuture jsonArrinsert(K key, String path, long index, V... jsons);
RedisFuture jsonArrlen(K key);
RedisFuture jsonArrlen(K key, String path);
RedisFuture jsonArrpop(K key);
RedisFuture jsonArrpop(K key, ArrpopOptions options);
RedisFuture jsonArrtrim(K key, String path, long start, long stop);
RedisFuture> jsonObjkeys(K key);
RedisFuture> jsonObjkeys(K key, String path);
RedisFuture jsonObjlen(K key);
RedisFuture jsonObjlen(K key, String path);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy