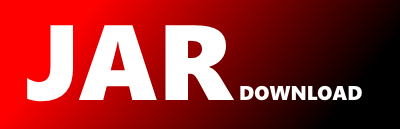
com.redis.lettucemod.util.IndexDefinitionParser Maven / Gradle / Ivy
package com.redis.lettucemod.util;
import java.util.Iterator;
import java.util.List;
import com.redis.lettucemod.search.CreateOptions;
import com.redis.lettucemod.search.CreateOptions.DataType;
import com.redis.lettucemod.search.Language;
public class IndexDefinitionParser {
private final CreateOptions.Builder options;
private final Iterator
© 2015 - 2024 Weber Informatics LLC | Privacy Policy