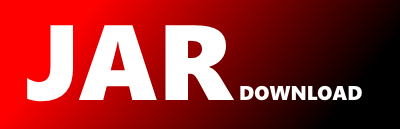
com.redis.lettucemod.timeseries.MRangeOptions Maven / Gradle / Ivy
package com.redis.lettucemod.timeseries;
import java.util.Optional;
import com.redis.lettucemod.protocol.TimeSeriesCommandKeyword;
import io.lettuce.core.CompositeArgument;
import io.lettuce.core.protocol.CommandArgs;
public class MRangeOptions extends AbstractRangeOptions {
private Optional> labels = Optional.empty();
private Filters filters = new Filters<>();
private Optional> groupBy = Optional.empty();
public MRangeOptions() {
}
private MRangeOptions(Builder builder) {
super(builder);
this.labels = builder.labels;
this.filters = builder.filters;
this.groupBy = builder.groupBy;
}
public Optional> getLabels() {
return labels;
}
public void setLabels(Optional> labels) {
this.labels = labels;
}
public Filters getFilters() {
return filters;
}
public void setFilters(Filters filters) {
this.filters = filters;
}
public Optional> getGroupBy() {
return groupBy;
}
public void setGroupBy(Optional> groupBy) {
this.groupBy = groupBy;
}
@SuppressWarnings("hiding")
@Override
public void build(CommandArgs args) {
super.build(args);
labels.ifPresent(l -> l.build(args));
filters.build(args);
groupBy.ifPresent(g -> g.build(args));
}
public static class GroupBy implements CompositeArgument {
private final K label;
private final Reducer reducer;
private GroupBy(K label, Reducer reducer) {
this.label = label;
this.reducer = reducer;
}
@SuppressWarnings("unchecked")
@Override
public void build(CommandArgs args) {
args.add(TimeSeriesCommandKeyword.GROUPBY);
args.addKey((L) label);
args.add(TimeSeriesCommandKeyword.REDUCE);
args.add(reducer.name());
}
public static GroupBy of(K label, Reducer reducer) {
return new GroupBy<>(label, reducer);
}
}
public enum Reducer {
SUM, MIN, MAX
}
@SuppressWarnings("unchecked")
public static Builder filters(V... filters) {
return new Builder<>(filters);
}
@SuppressWarnings("unchecked")
public static class Builder extends AbstractRangeOptions.Builder> {
private Optional> labels = Optional.empty();
private Filters filters = new Filters<>();
private Optional> groupBy = Optional.empty();
public Builder(V... filters) {
this.filters = Filters.of(filters);
}
public Builder filters(V... filters) {
this.filters = Filters.of(filters);
return this;
}
public Builder withLabels() {
this.labels = Optional.of(new Labels<>());
return this;
}
public Builder selectedLabels(K... labels) {
this.labels = Optional.of(Labels.of(labels));
return this;
}
public Builder groupBy(GroupBy groupBy) {
this.groupBy = Optional.of(groupBy);
return this;
}
public MRangeOptions build() {
return new MRangeOptions<>(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy