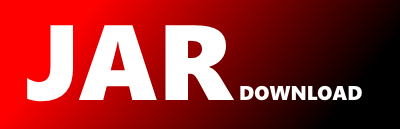
com.redis.spring.batch.reader.ListToKeyValue Maven / Gradle / Ivy
package com.redis.spring.batch.reader;
import java.util.Iterator;
import java.util.List;
import java.util.function.Function;
import com.redis.spring.batch.KeyValue;
import com.redis.spring.batch.util.CodecUtils;
import io.lettuce.core.codec.RedisCodec;
public class ListToKeyValue implements Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy