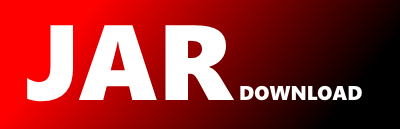
com.redis.spring.batch.util.CodecUtils Maven / Gradle / Ivy
package com.redis.spring.batch.util;
import java.util.function.Function;
import io.lettuce.core.codec.ByteArrayCodec;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.codec.StringCodec;
public abstract class CodecUtils {
private CodecUtils() {
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Function stringKeyFunction(RedisCodec codec) {
if (codec instanceof StringCodec) {
return (Function) Function.identity();
}
return key -> codec.decodeKey(StringCodec.UTF8.encodeKey(key));
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Function toStringKeyFunction(RedisCodec codec) {
if (codec instanceof StringCodec) {
return (Function) Function.identity();
}
return key -> StringCodec.UTF8.decodeKey(codec.encodeKey(key));
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Function stringValueFunction(RedisCodec, V> codec) {
if (codec instanceof StringCodec) {
return (Function) Function.identity();
}
return value -> codec.decodeValue(StringCodec.UTF8.encodeValue(value));
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Function toStringValueFunction(RedisCodec, V> codec) {
if (codec instanceof StringCodec) {
return (Function) Function.identity();
}
return value -> StringCodec.UTF8.decodeValue(codec.encodeValue(value));
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Function byteArrayKeyFunction(RedisCodec codec) {
if (codec instanceof ByteArrayCodec) {
return (Function) Function.identity();
}
return key -> codec.decodeKey(ByteArrayCodec.INSTANCE.encodeKey(key));
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Function toByteArrayKeyFunction(RedisCodec codec) {
if (codec instanceof ByteArrayCodec) {
return (Function) Function.identity();
}
return key -> ByteArrayCodec.INSTANCE.decodeKey(codec.encodeKey(key));
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Function byteArrayValueFunction(RedisCodec, V> codec) {
if (codec instanceof ByteArrayCodec) {
return (Function) Function.identity();
}
return value -> codec.decodeValue(ByteArrayCodec.INSTANCE.encodeValue(value));
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Function toByteArrayValueFunction(RedisCodec, V> codec) {
if (codec instanceof ByteArrayCodec) {
return (Function) Function.identity();
}
return value -> ByteArrayCodec.INSTANCE.decodeValue(codec.encodeValue(value));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy