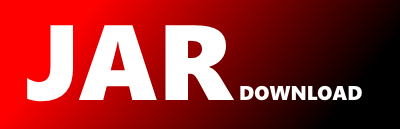
com.redis.spring.batch.util.KeyComparison Maven / Gradle / Ivy
package com.redis.spring.batch.util;
import java.util.Objects;
import com.redis.spring.batch.KeyValue;
public class KeyComparison {
public enum Status {
OK, // No difference
MISSING, // Key missing in target database
TYPE, // Type mismatch
TTL, // TTL mismatch
VALUE // Value mismatch
}
private KeyValue source;
private KeyValue target;
private Status status;
public KeyComparison(KeyValue source, KeyValue target, Status status) {
this.source = source;
this.target = target;
this.status = status;
}
public KeyValue getSource() {
return source;
}
public void setSource(KeyValue source) {
this.source = source;
}
public KeyValue getTarget() {
return target;
}
public void setTarget(KeyValue target) {
this.target = target;
}
public Status getStatus() {
return status;
}
public void setStatus(Status status) {
this.status = status;
}
@Override
public int hashCode() {
final int prime = 31;
int result = super.hashCode();
result = prime * result + Objects.hash(source, status, target);
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (!super.equals(obj))
return false;
if (getClass() != obj.getClass())
return false;
KeyComparison other = (KeyComparison) obj;
return Objects.equals(source, other.source) && status == other.status && Objects.equals(target, other.target);
}
@Override
public String toString() {
return "KeyComparison [source=" + source + ", target=" + target + ", status=" + status + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy