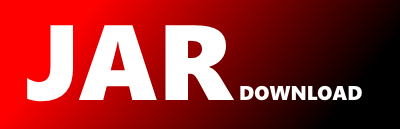
com.redis.spring.batch.util.CodecUtils Maven / Gradle / Ivy
The newest version!
package com.redis.spring.batch.util;
import java.nio.ByteBuffer;
import java.util.function.Function;
import io.lettuce.core.codec.ByteArrayCodec;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.codec.StringCodec;
public abstract class CodecUtils {
public static final StringCodec STRING_CODEC = StringCodec.UTF8;
public static final ByteArrayCodec BYTE_ARRAY_CODEC = ByteArrayCodec.INSTANCE;
private CodecUtils() {
}
public static Function stringKeyFunction(RedisCodec codec) {
Function encode = STRING_CODEC::encodeKey;
return encode.andThen(codec::decodeKey);
}
public static Function toStringKeyFunction(RedisCodec codec) {
Function encode = codec::encodeKey;
return encode.andThen(STRING_CODEC::decodeKey);
}
public static Function stringValueFunction(RedisCodec, V> codec) {
Function encode = STRING_CODEC::encodeValue;
return encode.andThen(codec::decodeValue);
}
public static Function toStringValueFunction(RedisCodec, V> codec) {
Function encode = codec::encodeValue;
return encode.andThen(STRING_CODEC::decodeValue);
}
public static Function byteArrayKeyFunction(RedisCodec codec) {
Function encode = BYTE_ARRAY_CODEC::encodeKey;
return encode.andThen(codec::decodeKey);
}
public static Function toByteArrayKeyFunction(RedisCodec codec) {
Function encode = codec::encodeKey;
return encode.andThen(BYTE_ARRAY_CODEC::decodeKey);
}
public static Function byteArrayValueFunction(RedisCodec, V> codec) {
Function encode = BYTE_ARRAY_CODEC::encodeValue;
return encode.andThen(codec::decodeValue);
}
public static Function toByteArrayValueFunction(RedisCodec, V> codec) {
Function encode = codec::encodeValue;
return encode.andThen(BYTE_ARRAY_CODEC::decodeValue);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy