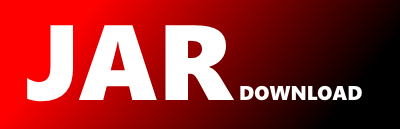
com.redis.spring.batch.util.Predicates Maven / Gradle / Ivy
The newest version!
package com.redis.spring.batch.util;
import java.util.Arrays;
import java.util.Collection;
import java.util.function.Function;
import java.util.function.IntPredicate;
import java.util.function.Predicate;
import java.util.function.ToIntFunction;
import java.util.regex.Pattern;
import java.util.stream.Stream;
import org.springframework.util.Assert;
import org.springframework.util.CollectionUtils;
import org.springframework.util.StringUtils;
import com.hrakaroo.glob.GlobPattern;
import com.redis.spring.batch.common.Range;
import io.lettuce.core.cluster.SlotHash;
import io.lettuce.core.codec.RedisCodec;
public interface Predicates {
@SuppressWarnings("unchecked")
static Predicate and(Predicate... predicates) {
return and(Arrays.asList(predicates));
}
static Predicate and(Collection> predicates) {
if (CollectionUtils.isEmpty(predicates)) {
return isTrue();
}
if (predicates.size() == 1) {
return predicates.iterator().next();
}
return and(predicates.stream());
}
static Predicate and(Stream> predicates) {
return predicates.reduce(isTrue(), Predicate::and);
}
@SuppressWarnings("unchecked")
static Predicate or(Predicate... predicates) {
return or(Arrays.asList(predicates));
}
static Predicate or(Collection> predicates) {
if (CollectionUtils.isEmpty(predicates)) {
return isTrue();
}
if (predicates.size() == 1) {
return predicates.iterator().next();
}
return or(predicates.stream());
}
static Predicate or(Stream> predicates) {
return predicates.reduce(isFalse(), Predicate::or);
}
static IntPredicate intAnd(IntPredicate... predicates) {
return intAnd(Arrays.asList(predicates));
}
static IntPredicate intAnd(Collection predicates) {
if (CollectionUtils.isEmpty(predicates)) {
return intIsTrue();
}
return intAnd(predicates.stream());
}
static IntPredicate intAnd(Stream predicates) {
return predicates.reduce(intIsTrue(), IntPredicate::and);
}
static IntPredicate intOr(IntPredicate... predicates) {
return intOr(Arrays.asList(predicates));
}
static IntPredicate intOr(Collection predicates) {
if (CollectionUtils.isEmpty(predicates)) {
return intIsTrue();
}
return intOr(predicates.stream());
}
static IntPredicate intOr(Stream predicates) {
return predicates.reduce(intIsFalse(), IntPredicate::or);
}
static Predicate intMap(ToIntFunction function, IntPredicate predicate) {
return k -> predicate.test(function.applyAsInt(k));
}
static Predicate map(Function function, Predicate predicate) {
return s -> predicate.test(function.apply(s));
}
static Predicate slots(Collection ranges) {
return slots(CodecUtils.STRING_CODEC, ranges);
}
static Predicate slots(RedisCodec codec, Collection ranges) {
if (CollectionUtils.isEmpty(ranges)) {
return isTrue();
}
return slots(codec, ranges.stream());
}
static Predicate slots(RedisCodec codec, Range... ranges) {
return slots(codec, Arrays.asList(ranges));
}
static Predicate slots(Range... ranges) {
return slots(CodecUtils.STRING_CODEC, ranges);
}
static Predicate slots(RedisCodec codec, Stream ranges) {
ToIntFunction slot = k -> SlotHash.getSlot(codec.encodeKey(k));
IntPredicate predicate = intAnd(ranges.map(Range::asPredicate).toArray(IntPredicate[]::new));
return intMap(slot, predicate);
}
static Predicate regex(String regex) {
return Pattern.compile(regex).asPredicate();
}
/**
* A {@link Predicate} that yields always {@code true}.
*
* @return a {@link Predicate} that yields always {@code true}.
*/
static Predicate isTrue() {
return is(true);
}
static IntPredicate intIsTrue() {
return intIs(true);
}
/**
* A {@link Predicate} that yields always {@code false}.
*
* @return a {@link Predicate} that yields always {@code false}.
*/
static Predicate isFalse() {
return is(false);
}
static IntPredicate intIsFalse() {
return intIs(false);
}
/**
* Returns a {@link Predicate} that represents the logical negation of {@code predicate}.
*
* @return a {@link Predicate} that represents the logical negation of {@code predicate}.
*/
static Predicate negate(Predicate predicate) {
Assert.notNull(predicate, "Predicate must not be null");
return predicate.negate();
}
static IntPredicate intNegate(IntPredicate predicate) {
Assert.notNull(predicate, "Predicate must not be null");
return predicate.negate();
}
static Predicate glob(String match) {
if (!StringUtils.hasLength(match)) {
return isTrue();
}
return GlobPattern.compile(match)::matches;
}
static Predicate is(boolean value) {
return t -> value;
}
static IntPredicate intIs(boolean value) {
return t -> value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy