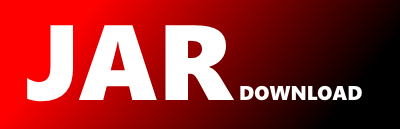
com.redis.spring.batch.writer.operation.ReplicaWait Maven / Gradle / Ivy
The newest version!
package com.redis.spring.batch.writer.operation;
import java.text.MessageFormat;
import java.time.Duration;
import org.springframework.batch.item.Chunk;
import com.redis.spring.batch.common.Operation;
import io.lettuce.core.RedisCommandExecutionException;
import io.lettuce.core.RedisFuture;
import io.lettuce.core.api.async.BaseRedisAsyncCommands;
import io.lettuce.core.cluster.PipelinedRedisFuture;
public class ReplicaWait implements Operation {
private final Operation delegate;
private final int replicas;
private final long timeout;
public ReplicaWait(Operation delegate, int replicas, Duration timeout) {
this.delegate = delegate;
this.replicas = replicas;
this.timeout = timeout.toMillis();
}
@SuppressWarnings({ "unchecked", "rawtypes" })
@Override
public void execute(BaseRedisAsyncCommands commands, Chunk extends T> items,
Chunk> outputs) {
delegate.execute(commands, items, outputs);
RedisFuture waitFuture = commands.waitForReplication(replicas, timeout);
outputs.add((RedisFuture) new PipelinedRedisFuture<>(waitFuture.thenAccept(this::checkReplicas)));
}
private void checkReplicas(Long actual) {
if (actual == null || actual < replicas) {
throw new RedisCommandExecutionException(errorMessage(actual));
}
}
private String errorMessage(Long actual) {
return MessageFormat.format("Insufficient replication level ({0}/{1})", actual, replicas);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy