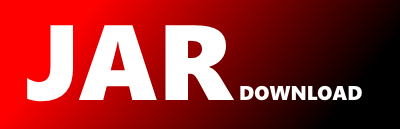
io.rebloom.client.cf.Cuckoo Maven / Gradle / Ivy
package io.rebloom.client.cf;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.stream.Stream;
/**
* Interface for RedisBloom Cuckoo Filter Commands
*
* @see RedisBloom
* Cuckoo Filter Documentation
*/
public interface Cuckoo {
/**
* CF.RESERVE Creates a Cuckoo Filter under key with the given parameters
*
* @param key The name of the filter
* @param options An instance of CFReserveOptions containing the options
* (CAPACITY/BUCKESIZE/MAXITERATIONS/EXPANSION)
*/
void cfCreate(String key, CFReserveOptions options);
/**
* CF.RESERVE Creates a Cuckoo Filter under key with a single sub-filter for the
* initial capacity
*
* @param key The key under which the filter is found
* @param capacity Estimated capacity for the filter
*/
void cfCreate(String key, long capacity);
/**
* CF.ADD Adds an item to the cuckoo filter, creating the filter if it does not
* exist
*
* @param key The name of the filter
* @param item The item to add
* @return true on success, false otherwise
*/
boolean cfAdd(String key, String item);
/**
* CF.ADDNX Adds an item to the cuckoo filter, only if it does not exist yet
*
* @param key The name of the filter
* @param item The item to add
* @return true if the item was added to the filter, false if the item already
* exists.
*/
boolean cfAddNx(String key, String item);
/**
* CF.INSERT Adds one or more items to a cuckoo filter, creating it if it does
* not exist yet.
*
* @param key The name of the filter
* @param items One or more items to add
* @return true if the item was successfully inserted, false if an error
* occurred
*/
List cfInsert(String key, String... items);
/**
* CF.INSERT Adds one or more items to a cuckoo filter, using the passed
* options
*
* @param key The name of the filter
* @param options An instance of CFInsertOptions containing the options
* (CAPACITY/NOCREATE)
* @param items One or more items to add
* @return true if the item was successfully inserted, false if an error
* occurred
*/
List cfInsert(String key, CFInsertOptions options, String... items);
/**
* CF.INSERTNX Adds one or more items to a cuckoo filter, only if it does not
* exist yet
*
* @param key The name of the filter
* @param items One or more items to add
* @return true if the item was added to the filter, false if the item already
* exists.
*/
List cfInsertNx(String key, String... items);
/**
* CF.INSERTNX Adds one or more items to a cuckoo filter, using the passed
* options
*
* @param key The name of the filter
* @param options An instance of CFInsertOptions containing the options
* (CAPACITY/NOCREATE)
* @param items One or more items to add
* @return true if the item was added to the filter, false if the item already
* exists.
*/
List cfInsertNx(String key, CFInsertOptions options, String... items);
/**
* CF.EXISTS Check if an item exists in a Cuckoo Filter
*
* @param key The name of the filter
* @param item The item to check for
* @return false if the item certainly does not exist, true if the item may
* exist.
*/
boolean cfExists(String key, String item);
/**
* CF.DEL Deletes an item once from the filter. If the item exists only once, it
* will be removed from the filter. If the item was added multiple times, it
* will still be present.
*
* @param key The name of the filter
* @param item The item to delete from the filter
* @return true if the item has been deleted, false if the item was not found.
*/
boolean cfDel(String key, String item);
/**
* CF.COUNT Returns the number of times an item may be in the filter.
*
* @param key The name of the filter
* @param item The item to count
* @return The number of times the item exists in the filter
*/
long cfCount(String key, String item);
/**
* CF.SCANDUMP Begins an incremental save of the cuckoo filter. This is useful
* for large cuckoo filters which cannot fit into the normal SAVE and RESTORE
* model.
*
* The Iterator is passed as input to the next invocation of SCANDUMP . If
* Iterator is 0, the iteration has completed.
*
* @param key Name of the filter
* @param iterator This is either 0, or the iterator from a previous invocation
* of this command
* @return a Map.Entry containing the Iterator and Data.
*/
Map.Entry cfScanDump(String key, long iterator);
/**
* CF.SCANDUMP Begins an incremental save of the cuckoo filter. This is useful
* for large cuckoo filters which cannot fit into the normal SAVE and RESTORE
* model.
*
* Returns an iterator over the Map.Entry containing the Iterator and Data in
* proper sequence.
*
* @param key Name of the filter
* @return An iterator over the Pair containing the chunks of byte[] representing the filter
*/
Iterator> cfScanDumpIterator(String key);
/**
* CF.SCANDUMP Begins an incremental save of the cuckoo filter. This is useful
* for large cuckoo filters which cannot fit into the normal SAVE and RESTORE
* model.
*
* Returns a sequential Stream with the Map.Entry containing the Iterator and
* Data as its source.
*
* @return A sequential Stream of Pair of iterator and data
*/
Stream> cfScanDumpStream(String key);
/**
* CF.LOADCHUNK Restores a filter previously saved using SCANDUMP . See the
* SCANDUMP command for example usage.
*
* @param key Name of the filter to restore
* @param iterAndData Pair of iterator and data
*/
void cfLoadChunk(String key, Map.Entry iterAndData);
/**
* CF.INFO Return information about filter
*
* @param key Name of the filter to restore
* @return A Map containing Size, Number of buckets, Number of filter, Number of
* items inserted, Number of items deleted, Bucket size, Expansion rate,
* Max iteration
*/
Map cfInfo(String key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy