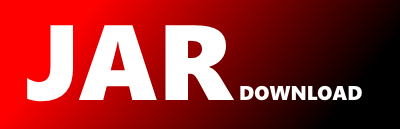
io.redisearch.AggregationResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jredisearch Show documentation
Show all versions of jredisearch Show documentation
Official client for Redis Search
The newest version!
package io.redisearch;
import io.redisearch.aggregation.Row;
import redis.clients.jedis.exceptions.JedisDataException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Created by mnunberg on 2/22/18.
*/
public class AggregationResult {
public final long totalResults;
private long cursorId = -1;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy