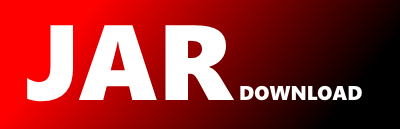
com.redislabs.redisgraph.impl.ResultSetImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jredisgraph Show documentation
Show all versions of jredisgraph Show documentation
Official client for Redis-Graph
package com.redislabs.redisgraph.impl;
import java.util.ArrayList;
import java.util.List;
import java.util.NoSuchElementException;
import java.util.stream.Collectors;
import com.redislabs.redisgraph.Record;
import com.redislabs.redisgraph.ResultSet;
import com.redislabs.redisgraph.Statistics;
import redis.clients.jedis.util.SafeEncoder;
public class ResultSetImpl implements ResultSet{
private final int totalResults;
private final List header;
private final List results;
private final Statistics statistics;
private int position = 0;
public ResultSetImpl(List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy