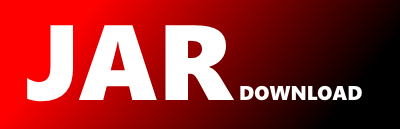
com.redislabs.redistimeseries.RedisTimeSeries Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jredistimeseries Show documentation
Show all versions of jredistimeseries Show documentation
Java Client for RedisTimeSeries.
package com.redislabs.redistimeseries;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import com.redislabs.redistimeseries.information.Info;
import com.redislabs.redistimeseries.information.Rule;
import redis.clients.jedis.BinaryClient;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.Protocol;
import redis.clients.jedis.exceptions.JedisDataException;
import redis.clients.jedis.util.Pool;
import redis.clients.jedis.util.SafeEncoder;
public class RedisTimeSeries {
private final Pool pool;
public RedisTimeSeries(Pool pool) {
this.pool = pool;
}
/**
* TS.CREATE key
*
* @param key
* @return
*/
public boolean create(String key){
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.CREATE, SafeEncoder.encode(key)).getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.CREATE key [RETENTION retentionSecs]
*
* @param key
* @param retentionSecs
* @return
*/
public boolean create(String key, long retentionSecs){
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.CREATE, SafeEncoder.encode(key), Keyword.RETENTION.getRaw(), Protocol.toByteArray(retentionSecs))
.getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.CREATE key [LABELS field value..]
*
* @param key
* @param maxSamplesPerChunk
* @return
*/
public boolean create(String key, Map labels){
try (Jedis conn = getConnection()) {
byte[][] args = new byte[1 + (labels==null ? 0 : 2*labels.size()+1)][];
int i=0;
args[i++] = SafeEncoder.encode(key);
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.CREATE, args).getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.CREATE key [RETENTION retentionSecs] [LABELS field value..]
*
* @param key
* @param retentionSecs
* @param maxSamplesPerChunk
* @return
*/
public boolean create(String key, long retentionSecs, Map labels){
try (Jedis conn = getConnection()) {
byte[][] args = new byte[3 + (labels==null ? 0 : 2*labels.size()+1)][];
int i=0;
args[i++] = SafeEncoder.encode(key);
args[i++] = Keyword.RETENTION.getRaw();
args[i++] = Protocol.toByteArray(retentionSecs);
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.CREATE, args).getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.CREATERULE sourceKey destKey AGGREGATION aggType bucketSizeSeconds
*
* @param sourceKey
* @param aggregation
* @param bucketSize
* @param destKey
* @return
*/
public boolean createRule(String sourceKey, Aggregation aggregation, long bucketSize, String destKey) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.CREATE_RULE, SafeEncoder.encode(sourceKey), SafeEncoder.encode(destKey),
Keyword.AGGREGATION.getRaw(), aggregation.getRaw(), Protocol.toByteArray(bucketSize))
.getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.DELETERULE SOURCE_KEY DEST_KEY
*
* @param sourceKey
* @param destKey
* @return
*/
public boolean deleteRule(String sourceKey, String destKey) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.DELETE_RULE, SafeEncoder.encode(sourceKey), SafeEncoder.encode(destKey))
.getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.ADD key timestamp value
*
* @param sourceKey
* @param timestamp
* @param value
* @return
*/
public boolean add(String sourceKey, long timestamp, double value) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.ADD, SafeEncoder.encode(sourceKey),
timestamp>0 ? Protocol.toByteArray(timestamp) : SafeEncoder.encode("*"), Protocol.toByteArray(value))
.getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionSecs]
*
* @param sourceKey
* @param timestamp
* @param value
* @param retentionSecs
* @return
*/
public boolean add(String sourceKey, long timestamp, double value, long retentionSecs) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.ADD, SafeEncoder.encode(sourceKey), timestamp>0 ? Protocol.toByteArray(timestamp) : SafeEncoder.encode("*"),
Protocol.toByteArray(value), Keyword.RETENTION.getRaw(), Protocol.toByteArray(retentionSecs))
.getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionSecs] [LABELS field value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param labels
* @return
*/
public boolean add(String sourceKey, long timestamp, double value, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = new byte[3 + (labels==null ? 0 : 2*labels.size()+1)][];
int i=0;
args[i++] = SafeEncoder.encode(sourceKey);
args[i++] = timestamp>0 ? Protocol.toByteArray(timestamp) : SafeEncoder.encode("*");
args[i++] = Protocol.toByteArray(value);
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.ADD, args).getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionSecs] [LABELS field value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param retentionSecs
* @param labels
* @return
*/
public boolean add(String sourceKey, long timestamp, double value, long retentionSecs, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = new byte[5 + (labels==null ? 0 : 2*labels.size()+1)][];
int i=0;
args[i++] = SafeEncoder.encode(sourceKey);
args[i++] = timestamp>0 ? Protocol.toByteArray(timestamp) : SafeEncoder.encode("*");
args[i++] = Protocol.toByteArray(value);
args[i++] = Keyword.RETENTION.getRaw();
args[i++] = Protocol.toByteArray(retentionSecs);
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.ADD, args).getStatusCodeReply().equals("OK");
} catch(JedisDataException ex ) {
throw new RedisTimeSeriesException(ex);
}
}
/**
* TS.RANGE key fromTimestamp toTimestamp
*
* @param key
* @param from
* @param to
* @return
*/
public Value[] range(String key, long from, long to) {
try (Jedis conn = getConnection()) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy