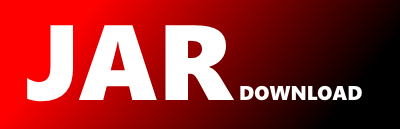
com.redislabs.redistimeseries.RedisTimeSeries Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jredistimeseries Show documentation
Show all versions of jredistimeseries Show documentation
Java Client for RedisTimeSeries.
package com.redislabs.redistimeseries;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import com.redislabs.redistimeseries.information.Info;
import com.redislabs.redistimeseries.information.Rule;
import redis.clients.jedis.BinaryClient;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
import redis.clients.jedis.Protocol;
import redis.clients.jedis.util.Pool;
import redis.clients.jedis.util.SafeEncoder;
public class RedisTimeSeries {
private static final byte[] STAR = SafeEncoder.encode("*");
private final Pool pool;
/**
* Create a new RedisTimeSeries client with default connection to local host
*/
public RedisTimeSeries() {
this("localhost", 6379);
}
/**
* Create a new RedisTimeSeries client
*
* @param host the redis host
* @param port the redis pot
*/
public RedisTimeSeries(String host, int port) {
this(host, port, 500, 100);
}
/**
* Create a new RedisTimeSeries client
*
* @param host the redis host
* @param port the redis pot
*/
public RedisTimeSeries(String host, int port, int timeout, int poolSize) {
this(host, port, timeout, poolSize, null);
}
/**
* Create a new RedisTimeSeries client
*
* @param host the redis host
* @param port the redis pot
* @param password the password for authentication in a password protected Redis server
*/
public RedisTimeSeries(String host, int port, int timeout, int poolSize, String password) {
this(new JedisPool(initPoolConfig(poolSize), host, port, timeout, password));
}
public RedisTimeSeries(Pool pool) {
this.pool = pool;
}
/**
* TS.CREATE key
*
* @param key
* @return
*/
public boolean create(String key){
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.CREATE, SafeEncoder.encode(key)).getStatusCodeReply().equals("OK");
}
}
/**
* TS.CREATE key [RETENTION retentionTime]
*
* @param key
* @param retentionTime
* @return
*/
public boolean create(String key, long retentionTime){
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.CREATE, SafeEncoder.encode(key), Keyword.RETENTION.getRaw(), Protocol.toByteArray(retentionTime))
.getStatusCodeReply().equals("OK");
}
}
/**
* TS.CREATE key [LABELS field value..]
*
* @param key
* @param labels
* @return
*/
public boolean create(String key, Map labels){
try (Jedis conn = getConnection()) {
byte[][] args = new byte[1 + (labels==null ? 0 : 2*labels.size()+1)][];
int i=0;
args[i++] = SafeEncoder.encode(key);
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.CREATE, args).getStatusCodeReply().equals("OK");
}
}
/**
* TS.CREATE key [RETENTION retentionTime] [LABELS field value..]
*
* @param key
* @param retentionTime
* @param labels
* @return
*/
public boolean create(String key, long retentionTime, Map labels){
return create(key, retentionTime, false, labels);
}
/**
* TS.CREATE key [RETENTION retentionTime] [UNCOMPRESSED] [LABELS field value..]
*
* @param key
* @param retentionTime
* @param uncompressed
* @param labels
* @return
*/
public boolean create(String key, long retentionTime, boolean uncompressed, Map labels){
try (Jedis conn = getConnection()) {
byte[][] args = new byte[3 + (labels==null ? 0 : 2*labels.size()+1) + (uncompressed?1:0)][];
int i=0;
args[i++] = SafeEncoder.encode(key);
args[i++] = Keyword.RETENTION.getRaw();
args[i++] = Protocol.toByteArray(retentionTime);
if(uncompressed) {
args[i++] = Keyword.UNCOMPRESSED.getRaw();
}
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.CREATE, args).getStatusCodeReply().equals("OK");
}
}
/**
* TS.ALTER key [LABELS label value..]
* @param key
* @param labels
* @return
*/
public boolean alter(String key, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = new byte[1 + (labels==null ? 0 : 2*labels.size()+1)][];
int i=0;
args[i++] = SafeEncoder.encode(key);
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.ALTER, args).getStatusCodeReply().equals("OK");
}
}
/**
* TS.ALTER key [RETENTION retentionTime] [LABELS label value..]
* @param key
* @param retentionTime
* @param labels
* @return
*/
public boolean alter(String key, long retentionTime, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = new byte[3 + (labels==null ? 0 : 2*labels.size()+1)][];
int i=0;
args[i++] = SafeEncoder.encode(key);
args[i++] = Keyword.RETENTION.getRaw();
args[i++] = Protocol.toByteArray(retentionTime);
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.ALTER, args).getStatusCodeReply().equals("OK");
}
}
/**
* TS.CREATERULE sourceKey destKey AGGREGATION aggType retentionTime
*
* @param sourceKey
* @param aggregation
* @param bucketSize
* @param destKey
* @return
*/
public boolean createRule(String sourceKey, Aggregation aggregation, long bucketSize, String destKey) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.CREATE_RULE, SafeEncoder.encode(sourceKey), SafeEncoder.encode(destKey),
Keyword.AGGREGATION.getRaw(), aggregation.getRaw(), Protocol.toByteArray(bucketSize))
.getStatusCodeReply().equals("OK");
}
}
/**
* TS.DELETERULE SOURCE_KEY DEST_KEY
*
* @param sourceKey
* @param destKey
* @return
*/
public boolean deleteRule(String sourceKey, String destKey) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.DELETE_RULE, SafeEncoder.encode(sourceKey), SafeEncoder.encode(destKey))
.getStatusCodeReply().equals("OK");
}
}
/**
* TS.ADD key * value
*
* @param sourceKey
* @param value
* @return
*/
public long add(String sourceKey, double value) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.ADD, SafeEncoder.encode(sourceKey),
STAR, Protocol.toByteArray(value))
.getIntegerReply();
}
}
/**
* TS.ADD key timestamp value
*
* @param sourceKey
* @param timestamp
* @param value
* @return
*/
public long add(String sourceKey, long timestamp, double value) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.ADD, SafeEncoder.encode(sourceKey),
timestamp>0 ? Protocol.toByteArray(timestamp) : STAR, Protocol.toByteArray(value))
.getIntegerReply();
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime]
*
* @param sourceKey
* @param timestamp
* @param value
* @param retentionTime
* @return
*/
public long add(String sourceKey, long timestamp, double value, long retentionTime) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.ADD, SafeEncoder.encode(sourceKey), timestamp>0 ? Protocol.toByteArray(timestamp) : STAR,
Protocol.toByteArray(value), Keyword.RETENTION.getRaw(), Protocol.toByteArray(retentionTime)).getIntegerReply();
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime] [LABELS field value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param labels
* @return
*/
public long add(String sourceKey, long timestamp, double value, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = new byte[3 + (labels==null ? 0 : 2*labels.size()+1)][];
int i=0;
args[i++] = SafeEncoder.encode(sourceKey);
args[i++] = timestamp>0 ? Protocol.toByteArray(timestamp) : STAR;
args[i++] = Protocol.toByteArray(value);
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.ADD, args).getIntegerReply();
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime] [LABELS field value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param retentionTime
* @param labels
* @return
*/
public long add(String sourceKey, long timestamp, double value, long retentionTime, Map labels) {
return add(sourceKey, timestamp, value, retentionTime, false, labels);
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime] UNCOMPRESSED [LABELS field value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param retentionTime
* @param uncompressed
* @param labels
* @return
*/
public long add(String sourceKey, long timestamp, double value, long retentionTime, boolean uncompressed, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = new byte[5 + (labels==null ? 0 : 2*labels.size()+1) + (uncompressed?1:0)][];
int i=0;
args[i++] = SafeEncoder.encode(sourceKey);
args[i++] = timestamp>0 ? Protocol.toByteArray(timestamp) : STAR;
args[i++] = Protocol.toByteArray(value);
args[i++] = Keyword.RETENTION.getRaw();
args[i++] = Protocol.toByteArray(retentionTime);
if(uncompressed) {
args[i++] = Keyword.UNCOMPRESSED.getRaw();
}
if(labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for(Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return sendCommand(conn, Command.ADD, args).getIntegerReply();
}
}
/**
* TS.MADD key timestamp value [key timestamp value ...]
*
* @param measurements
* @return
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy