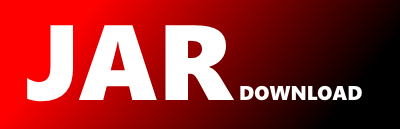
com.redislabs.redistimeseries.RedisTimeSeries Maven / Gradle / Ivy
package com.redislabs.redistimeseries;
import com.redislabs.redistimeseries.information.Info;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import redis.clients.jedis.BinaryClient;
import redis.clients.jedis.Client;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
import redis.clients.jedis.Protocol;
import redis.clients.jedis.util.Pool;
import redis.clients.jedis.util.SafeEncoder;
public class RedisTimeSeries implements AutoCloseable {
private static final byte[] STAR = SafeEncoder.encode("*");
protected static final byte[] PLUS = SafeEncoder.encode("+");
protected static final byte[] MINUS = SafeEncoder.encode("-");
private final Pool pool;
/** Create a new RedisTimeSeries client with default connection to local host */
public RedisTimeSeries() {
this("localhost", 6379);
}
public RedisTimeSeries(String host) {
this(new JedisPool(host));
}
/**
* Create a new RedisTimeSeries client
*
* @param host the redis host
* @param port the redis pot
*/
public RedisTimeSeries(String host, int port) {
this(host, port, 500, 100);
}
/**
* Create a new RedisTimeSeries client
*
* @param host the redis host
* @param port the redis pot
*/
public RedisTimeSeries(String host, int port, int timeout, int poolSize) {
this(host, port, timeout, poolSize, null);
}
/**
* Create a new RedisTimeSeries client
*
* @param host the redis host
* @param port the redis pot
* @param password the password for authentication in a password protected Redis server
*/
public RedisTimeSeries(String host, int port, int timeout, int poolSize, String password) {
this(new JedisPool(initPoolConfig(poolSize), host, port, timeout, password));
}
public RedisTimeSeries(Pool pool) {
this.pool = pool;
}
/**
* TS.CREATE key
*
* @param key
* @return
*/
public boolean create(String key) {
try (Jedis conn = getConnection()) {
byte[][] args = tsCreateArgs(key, null, false, null, null, null);
return sendCommand(conn, Command.CREATE, args).getStatusCodeReply().equals("OK");
}
}
/**
* TS.CREATE key [RETENTION retentionTime]
*
* @param key
* @param retentionTime
* @return
*/
public boolean create(String key, long retentionTime) {
return create(key, retentionTime, false, null);
}
/**
* TS.CREATE key [LABELS field value..]
*
* @param key
* @param labels
* @return
*/
public boolean create(String key, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = tsCreateArgs(key, null, false, null, null, labels);
return sendCommand(conn, Command.CREATE, args).getStatusCodeReply().equals("OK");
}
}
/**
* TS.CREATE key [RETENTION retentionTime] [LABELS field value..]
*
* @param key
* @param retentionTime
* @param labels
* @return
*/
public boolean create(String key, long retentionTime, Map labels) {
return create(key, retentionTime, false, labels);
}
/**
* TS.CREATE key [RETENTION retentionTime] [UNCOMPRESSED] [LABELS field value..]
*
* @param key
* @param retentionTime
* @param uncompressed
* @param labels
* @return
*/
public boolean create(
String key, long retentionTime, boolean uncompressed, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = tsCreateArgs(key, retentionTime, uncompressed, null, null, labels);
return sendCommand(conn, Command.CREATE, args).getStatusCodeReply().equals("OK");
}
}
/**
* TS.CREATE key [RETENTION retentionTime] [UNCOMPRESSED] [CHUNK_SIZE size] [DUPLICATE_POLICY
* policy] [LABELS label value..]
*
* @param key
* @param retentionTime
* @param uncompressed
* @param labels
* @param chunkSize
* @param duplicatePolicy
* @return
*/
public boolean create(
String key,
long retentionTime,
boolean uncompressed,
long chunkSize,
DuplicatePolicy duplicatePolicy,
Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args =
tsCreateArgs(key, retentionTime, uncompressed, chunkSize, duplicatePolicy, labels);
return sendCommand(conn, Command.CREATE, args).getStatusCodeReply().equals("OK");
}
}
private static byte[][] tsCreateArgs(
String key,
Long retentionTime,
boolean uncompressed,
Long chunkSize,
DuplicatePolicy duplicatePolicy,
Map labels) {
byte[][] args =
new byte
[1
+ (labels == null ? 0 : 2 * labels.size() + 1)
+ (retentionTime != null ? 2 : 0)
+ (duplicatePolicy != null ? 2 : 0)
+ (chunkSize != null ? 2 : 0)
+ (uncompressed ? 1 : 0)]
[];
int i = 0;
args[i++] = SafeEncoder.encode(key);
if (retentionTime != null) {
args[i++] = Keyword.RETENTION.getRaw();
args[i++] = Protocol.toByteArray(retentionTime.longValue());
}
if (uncompressed) {
args[i++] = Keyword.UNCOMPRESSED.getRaw();
}
if (chunkSize != null) {
args[i++] = Keyword.CHUNK_SIZE.getRaw();
args[i++] = Protocol.toByteArray(chunkSize.longValue());
}
if (duplicatePolicy != null) {
args[i++] = Keyword.DUPLICATE_POLICY.getRaw();
args[i++] = duplicatePolicy.getRaw();
}
if (labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for (Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return args;
}
/**
* TS.CREATE key [RETENTION retentionTime] [UNCOMPRESSED] [CHUNK_SIZE size] [DUPLICATE_POLICY
* policy] [LABELS label value..]
*
* @param key
* @param createParams
* @return
*/
public boolean create(String key, CreateParams createParams) {
try (Jedis conn = getConnection()) {
List params = new ArrayList<>();
params.add(SafeEncoder.encode(key));
createParams.addOptionalParams(params);
return sendCommand(conn, Command.CREATE, params.toArray(new byte[params.size()][]))
.getStatusCodeReply()
.equals("OK");
}
}
/**
* TS.DEL key fromTimestamp toTimestamp
*
* @param key
* @param from timestamp from
* @param to timestamp to
* @return
*/
public long del(String key, long from, long to) {
try (Jedis conn = getConnection()) {
return sendCommand(conn, Command.DEL, key, Long.toString(from), Long.toString(to))
.getIntegerReply();
}
}
/**
* TS.ALTER key [LABELS label value..]
*
* @param key
* @param labels
* @return
*/
public boolean alter(String key, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = tsAlterArgs(key, null, labels);
return sendCommand(conn, Command.ALTER, args).getStatusCodeReply().equals("OK");
}
}
/**
* TS.ALTER key [RETENTION retentionTime] [LABELS label value..]
*
* @param key
* @param retentionTime
* @param labels
* @return
*/
public boolean alter(String key, long retentionTime, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = tsAlterArgs(key, retentionTime, labels);
return sendCommand(conn, Command.ALTER, args).getStatusCodeReply().equals("OK");
}
}
private static byte[][] tsAlterArgs(String key, Long retentionTime, Map labels) {
byte[][] args =
new byte[1 + (retentionTime != null ? 2 : 0) + (labels == null ? 0 : 2 * labels.size() + 1)]
[];
int i = 0;
args[i++] = SafeEncoder.encode(key);
if (retentionTime != null) {
args[i++] = Keyword.RETENTION.getRaw();
args[i++] = Protocol.toByteArray(retentionTime.longValue());
}
if (labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for (Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return args;
}
/**
* TS.CREATERULE sourceKey destKey AGGREGATION aggType retentionTime
*
* @param sourceKey
* @param aggregation
* @param bucketSize
* @param destKey
* @return
*/
public boolean createRule(
String sourceKey, Aggregation aggregation, long bucketSize, String destKey) {
try (Jedis conn = getConnection()) {
return sendCommand(
conn,
Command.CREATE_RULE,
SafeEncoder.encode(sourceKey),
SafeEncoder.encode(destKey),
Keyword.AGGREGATION.getRaw(),
aggregation.getRaw(),
Protocol.toByteArray(bucketSize))
.getStatusCodeReply()
.equals("OK");
}
}
/**
* TS.DELETERULE SOURCE_KEY DEST_KEY
*
* @param sourceKey
* @param destKey
* @return
*/
public boolean deleteRule(String sourceKey, String destKey) {
try (Jedis conn = getConnection()) {
return sendCommand(
conn, Command.DELETE_RULE, SafeEncoder.encode(sourceKey), SafeEncoder.encode(destKey))
.getStatusCodeReply()
.equals("OK");
}
}
/**
* TS.ADD key * value
*
* @param sourceKey
* @param value
* @return
*/
public long add(String sourceKey, double value) {
try (Jedis conn = getConnection()) {
byte[][] args = tsAddArgs(sourceKey, null, value, null, false, null, null, null);
return sendCommand(conn, Command.ADD, args).getIntegerReply();
}
}
/**
* TS.ADD key timestamp value
*
* @param sourceKey
* @param timestamp
* @param value
* @return
*/
public long add(String sourceKey, long timestamp, double value) {
try (Jedis conn = getConnection()) {
byte[][] args = tsAddArgs(sourceKey, timestamp, value, null, false, null, null, null);
return sendCommand(conn, Command.ADD, args).getIntegerReply();
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime]
*
* @param sourceKey
* @param timestamp
* @param value
* @param retentionTime
* @return
*/
public long add(String sourceKey, long timestamp, double value, long retentionTime) {
return add(sourceKey, timestamp, value, retentionTime, false, null);
}
/**
* TS.ADD key * value [RETENTION retentionTime]
*
* @param sourceKey
* @param value
* @param retentionTime
* @return
*/
public long add(String sourceKey, double value, long retentionTime) {
try (Jedis conn = getConnection()) {
byte[][] args = tsAddArgs(sourceKey, null, value, retentionTime, false, null, null, null);
return sendCommand(conn, Command.ADD, args).getIntegerReply();
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime] [LABELS field value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param labels
* @return
*/
public long add(String sourceKey, long timestamp, double value, Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args = tsAddArgs(sourceKey, timestamp, value, null, false, null, null, labels);
return sendCommand(conn, Command.ADD, args).getIntegerReply();
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime] [LABELS field value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param retentionTime
* @param labels
* @return
*/
public long add(
String sourceKey,
long timestamp,
double value,
long retentionTime,
Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args =
tsAddArgs(sourceKey, timestamp, value, retentionTime, false, null, null, labels);
return sendCommand(conn, Command.ADD, args).getIntegerReply();
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime] UNCOMPRESSED [LABELS field value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param retentionTime
* @param uncompressed
* @param labels
* @return
*/
public long add(
String sourceKey,
long timestamp,
double value,
long retentionTime,
boolean uncompressed,
Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args =
tsAddArgs(sourceKey, timestamp, value, retentionTime, uncompressed, null, null, labels);
return sendCommand(conn, Command.ADD, args).getIntegerReply();
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime] UNCOMPRESSED [CHUNK_SIZE size]
* [ON_DUPLICATE policy] [LABELS label value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param retentionTime
* @param chunkSize
* @param duplicatePolicy
* @param uncompressed
* @param labels
* @return
*/
public long add(
String sourceKey,
long timestamp,
double value,
long retentionTime,
boolean uncompressed,
long chunkSize,
DuplicatePolicy duplicatePolicy,
Map labels) {
try (Jedis conn = getConnection()) {
byte[][] args =
tsAddArgs(
sourceKey,
timestamp,
value,
retentionTime,
uncompressed,
chunkSize,
duplicatePolicy,
labels);
return sendCommand(conn, Command.ADD, args).getIntegerReply();
}
}
private static byte[][] tsAddArgs(
String sourceKey,
Long timestamp,
double value,
Long retentionTime,
boolean uncompressed,
Long chunkSize,
DuplicatePolicy duplicatePolicy,
Map labels) {
byte[][] args =
new byte
[3
+ (retentionTime != null ? 2 : 0)
+ (uncompressed ? 1 : 0)
+ (chunkSize != null ? 2 : 0)
+ (duplicatePolicy != null ? 2 : 0)
+ (labels == null ? 0 : 2 * labels.size() + 1)]
[];
int i = 0;
args[i++] = SafeEncoder.encode(sourceKey);
args[i++] = timestamp != null ? Protocol.toByteArray(timestamp) : STAR;
args[i++] = Protocol.toByteArray(value);
if (retentionTime != null) {
args[i++] = Keyword.RETENTION.getRaw();
args[i++] = Protocol.toByteArray(retentionTime);
}
if (uncompressed) {
args[i++] = Keyword.UNCOMPRESSED.getRaw();
}
if (chunkSize != null) {
args[i++] = Keyword.CHUNK_SIZE.getRaw();
args[i++] = Protocol.toByteArray(chunkSize);
}
if (duplicatePolicy != null) {
args[i++] = Keyword.ON_DUPLICATE.getRaw();
args[i++] = duplicatePolicy.getRaw();
}
if (labels != null) {
args[i++] = Keyword.LABELS.getRaw();
for (Entry e : labels.entrySet()) {
args[i++] = SafeEncoder.encode(e.getKey());
args[i++] = SafeEncoder.encode(e.getValue());
}
}
return args;
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime] [UNCOMPRESSED] [CHUNK_SIZE size]
* [ON_DUPLICATE policy] [LABELS label value..]
*
* @param sourceKey
* @param value
* @param createParams
* @return
*/
public long add(String sourceKey, double value, CreateParams createParams) {
try (Jedis conn = getConnection()) {
List params = new ArrayList<>();
params.add(SafeEncoder.encode(sourceKey));
params.add(Protocol.BYTES_ASTERISK);
params.add(Protocol.toByteArray(value));
createParams.addOptionalParams(params);
return sendCommand(conn, Command.ADD, params.toArray(new byte[params.size()][]))
.getIntegerReply();
}
}
/**
* TS.ADD key timestamp value [RETENTION retentionTime] [UNCOMPRESSED] [CHUNK_SIZE size]
* [ON_DUPLICATE policy] [LABELS label value..]
*
* @param sourceKey
* @param timestamp
* @param value
* @param addParams
* @return
*/
public long add(String sourceKey, long timestamp, double value, CreateParams addParams) {
try (Jedis conn = getConnection()) {
List params = new ArrayList<>();
params.add(SafeEncoder.encode(sourceKey));
params.add(Protocol.toByteArray(timestamp));
params.add(Protocol.toByteArray(value));
addParams.addOptionalParams(params);
return sendCommand(conn, Command.ADD, params.toArray(new byte[params.size()][]))
.getIntegerReply();
}
}
/**
* TS.MADD key timestamp value [key timestamp value ...]
*
* @param measurements
* @return
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy