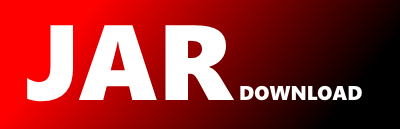
com.redislabs.lettusearch.output.AggregateOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lettusearch Show documentation
Show all versions of lettusearch Show documentation
Java client for RediSearch based on Lettuce
package com.redislabs.lettusearch.output;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import com.redislabs.lettusearch.aggregate.AggregateResults;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.output.CommandOutput;
import io.lettuce.core.output.MapOutput;
public class AggregateOutput> extends CommandOutput {
private final MapOutput nested;
private int mapCount = -1;
private final List counts = new ArrayList<>();
public AggregateOutput(RedisCodec codec, R results) {
super(codec, results);
nested = new MapOutput<>(codec);
}
public int getMapCount() {
return mapCount;
}
@Override
public void set(ByteBuffer bytes) {
nested.set(bytes);
}
@Override
public void complete(int depth) {
if (!counts.isEmpty()) {
int expectedSize = counts.get(0);
if (nested.get().size() == expectedSize) {
counts.remove(0);
output.add(new LinkedHashMap<>(nested.get()));
nested.get().clear();
}
}
}
@Override
public void set(long integer) {
output.setCount(integer);
}
@Override
public void multi(int count) {
nested.multi(count);
if (mapCount == -1) {
mapCount = count - 1;
} else {
// div 2 because of key value pair counts twice
counts.add(count / 2);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy