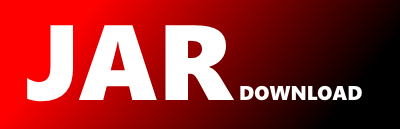
com.redislabs.mesclun.impl.RedisModulesAsyncCommandsImpl Maven / Gradle / Ivy
package com.redislabs.mesclun.impl;
import com.redislabs.mesclun.StatefulRedisModulesConnection;
import com.redislabs.mesclun.RedisModulesAsyncCommands;
import com.redislabs.mesclun.gears.*;
import com.redislabs.mesclun.gears.output.ExecutionResults;
import com.redislabs.mesclun.search.*;
import com.redislabs.mesclun.timeseries.Aggregation;
import com.redislabs.mesclun.timeseries.CreateOptions;
import com.redislabs.mesclun.timeseries.Label;
import com.redislabs.mesclun.timeseries.RedisTimeSeriesCommandBuilder;
import io.lettuce.core.RedisAsyncCommandsImpl;
import io.lettuce.core.RedisFuture;
import io.lettuce.core.codec.RedisCodec;
import java.util.List;
import java.util.Map;
@SuppressWarnings("unchecked")
public class RedisModulesAsyncCommandsImpl extends RedisAsyncCommandsImpl implements RedisModulesAsyncCommands {
private final StatefulRedisModulesConnection connection;
private final RedisGearsCommandBuilder gearsCommandBuilder;
private final RedisTimeSeriesCommandBuilder timeSeriesCommandBuilder;
private final RediSearchCommandBuilder searchCommandBuilder;
public RedisModulesAsyncCommandsImpl(StatefulRedisModulesConnection connection, RedisCodec codec) {
super(connection, codec);
this.connection = connection;
this.gearsCommandBuilder = new RedisGearsCommandBuilder<>(codec);
this.timeSeriesCommandBuilder = new RedisTimeSeriesCommandBuilder<>(codec);
this.searchCommandBuilder = new RediSearchCommandBuilder<>(codec);
}
@Override
public StatefulRedisModulesConnection getStatefulConnection() {
return connection;
}
@Override
public RedisFuture abortExecution(String id) {
return dispatch(gearsCommandBuilder.abortExecution(id));
}
@Override
public RedisFuture> configGet(K... keys) {
return dispatch(gearsCommandBuilder.configGet(keys));
}
@Override
public RedisFuture> configSet(Map map) {
return dispatch(gearsCommandBuilder.configSet(map));
}
@Override
public RedisFuture dropExecution(String id) {
return dispatch(gearsCommandBuilder.dropExecution(id));
}
@Override
public RedisFuture> dumpExecutions() {
return dispatch(gearsCommandBuilder.dumpExecutions());
}
@Override
public RedisFuture pyExecute(String function, V... requirements) {
return dispatch(gearsCommandBuilder.pyExecute(function, requirements));
}
@Override
public RedisFuture pyExecuteUnblocking(String function, V... requirements) {
return dispatch(gearsCommandBuilder.pyExecuteUnblocking(function, requirements));
}
@Override
public RedisFuture> trigger(String trigger, V... args) {
return dispatch(gearsCommandBuilder.trigger(trigger, args));
}
@Override
public RedisFuture unregister(String id) {
return dispatch(gearsCommandBuilder.unregister(id));
}
@Override
public RedisFuture> dumpRegistrations() {
return dispatch(gearsCommandBuilder.dumpRegistrations());
}
@Override
public RedisFuture getExecution(String id) {
return dispatch(gearsCommandBuilder.getExecution(id));
}
@Override
public RedisFuture getExecution(String id, ExecutionMode mode) {
return dispatch(gearsCommandBuilder.getExecution(id, mode));
}
@Override
public RedisFuture getResults(String id) {
return dispatch(gearsCommandBuilder.getResults(id));
}
@Override
public RedisFuture getResultsBlocking(String id) {
return dispatch(gearsCommandBuilder.getResultsBlocking(id));
}
@SuppressWarnings("unchecked")
@Override
public RedisFuture create(K key, Label... labels) {
return dispatch(timeSeriesCommandBuilder.create(key, null, labels));
}
@SuppressWarnings("unchecked")
@Override
public RedisFuture create(K key, CreateOptions options, Label... labels) {
return dispatch(timeSeriesCommandBuilder.create(key, options, labels));
}
@SuppressWarnings("unchecked")
@Override
public RedisFuture add(K key, long timestamp, double value, Label... labels) {
return dispatch(timeSeriesCommandBuilder.add(key, timestamp, value, null, labels));
}
@SuppressWarnings("unchecked")
@Override
public RedisFuture add(K key, long timestamp, double value, CreateOptions options, Label... labels) {
return dispatch(timeSeriesCommandBuilder.add(key, timestamp, value, options, labels));
}
@Override
public RedisFuture createRule(K sourceKey, K destKey, Aggregation aggregationType, long timeBucket) {
return dispatch(timeSeriesCommandBuilder.createRule(sourceKey, destKey, aggregationType, timeBucket));
}
@Override
public RedisFuture deleteRule(K sourceKey, K destKey) {
return dispatch(timeSeriesCommandBuilder.deleteRule(sourceKey, destKey));
}
@Override
public RedisFuture create(K index, Field... fields) {
return create(index, null, fields);
}
@Override
public RedisFuture create(K index, com.redislabs.mesclun.search.CreateOptions options, Field... fields) {
return dispatch(searchCommandBuilder.create(index, options, fields));
}
@Override
public RedisFuture dropIndex(K index) {
return dropIndex(index, false);
}
@Override
public RedisFuture dropIndex(K index, boolean deleteDocs) {
return dispatch(searchCommandBuilder.dropIndex(index, deleteDocs));
}
@Override
public RedisFuture> indexInfo(K index) {
return dispatch(searchCommandBuilder.info(index));
}
@Override
public RedisFuture> search(K index, V query) {
return dispatch(searchCommandBuilder.search(index, query, null));
}
@Override
public RedisFuture> search(K index, V query, SearchOptions options) {
return dispatch(searchCommandBuilder.search(index, query, options));
}
@Override
public RedisFuture> aggregate(K index, V query) {
return dispatch(searchCommandBuilder.aggregate(index, query, null));
}
@Override
public RedisFuture> aggregate(K index, V query, AggregateOptions options) {
return dispatch(searchCommandBuilder.aggregate(index, query, options));
}
@Override
public RedisFuture> aggregate(K index, V query, Cursor cursor) {
return dispatch(searchCommandBuilder.aggregate(index, query, cursor, null));
}
@Override
public RedisFuture> aggregate(K index, V query, Cursor cursor, AggregateOptions options) {
return dispatch(searchCommandBuilder.aggregate(index, query, cursor, options));
}
@Override
public RedisFuture> cursorRead(K index, long cursor) {
return dispatch(searchCommandBuilder.cursorRead(index, cursor, null));
}
@Override
public RedisFuture> cursorRead(K index, long cursor, long count) {
return dispatch(searchCommandBuilder.cursorRead(index, cursor, count));
}
@Override
public RedisFuture cursorDelete(K index, long cursor) {
return dispatch(searchCommandBuilder.cursorDelete(index, cursor));
}
@Override
public RedisFuture sugadd(K key, V string, double score) {
return dispatch(searchCommandBuilder.sugadd(key, string, score));
}
@Override
public RedisFuture sugadd(K key, V string, double score, SugaddOptions options) {
return dispatch(searchCommandBuilder.sugadd(key, string, score, options));
}
@Override
public RedisFuture>> sugget(K key, V prefix) {
return dispatch(searchCommandBuilder.sugget(key, prefix));
}
@Override
public RedisFuture>> sugget(K key, V prefix, SuggetOptions options) {
return dispatch(searchCommandBuilder.sugget(key, prefix, options));
}
@Override
public RedisFuture sugdel(K key, V string) {
return dispatch(searchCommandBuilder.sugdel(key, string));
}
@Override
public RedisFuture suglen(K key) {
return dispatch(searchCommandBuilder.suglen(key));
}
@Override
public RedisFuture alter(K index, Field field) {
return dispatch(searchCommandBuilder.alter(index, field));
}
@Override
public RedisFuture aliasAdd(K name, K index) {
return dispatch(searchCommandBuilder.aliasAdd(name, index));
}
@Override
public RedisFuture aliasDel(K name) {
return dispatch(searchCommandBuilder.aliasDel(name));
}
@Override
public RedisFuture aliasUpdate(K name, K index) {
return dispatch(searchCommandBuilder.aliasUpdate(name, index));
}
@Override
public RedisFuture> list() {
return dispatch(searchCommandBuilder.list());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy