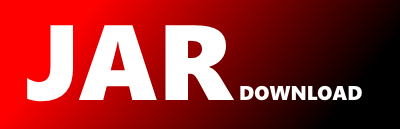
com.redislabs.mesclun.timeseries.RedisTimeSeriesCommandBuilder Maven / Gradle / Ivy
package com.redislabs.mesclun.timeseries;
import com.redislabs.mesclun.impl.RedisModulesCommandBuilder;
import com.redislabs.mesclun.timeseries.protocol.CommandKeyword;
import com.redislabs.mesclun.timeseries.protocol.CommandType;
import io.lettuce.core.codec.RedisCodec;
import io.lettuce.core.output.CommandOutput;
import io.lettuce.core.output.IntegerOutput;
import io.lettuce.core.output.StatusOutput;
import io.lettuce.core.protocol.Command;
import io.lettuce.core.protocol.CommandArgs;
/**
* Dedicated builder to build RedisTimeSeries commands.
*/
public class RedisTimeSeriesCommandBuilder extends RedisModulesCommandBuilder {
public RedisTimeSeriesCommandBuilder(RedisCodec codec) {
super(codec);
}
protected Command createCommand(CommandType type, CommandOutput output,
CommandArgs args) {
return new Command<>(type, output, args);
}
public Command create(K key, CreateOptions options, Label[] labels) {
CommandArgs args = args(key);
addOptions(args, options, labels);
return createCommand(CommandType.CREATE, new StatusOutput<>(codec), args);
}
public Command add(K key, long timestamp, double value, CreateOptions options, Label[] labels) {
CommandArgs args = args(key);
args.add(timestamp);
args.add(value);
addOptions(args, options, labels);
return createCommand(CommandType.ADD, new IntegerOutput<>(codec), args);
}
private void addOptions(CommandArgs args, CreateOptions options, Label[] labels) {
if (options != null) {
options.build(args);
}
if (labels != null && labels.length > 0) {
args.add(CommandKeyword.LABELS);
for (Label label : labels) {
args.addKey(label.getLabel());
args.addValue(label.getValue());
}
}
}
private CommandArgs args(K key) {
notNull(key, "key");
return new CommandArgs<>(codec).addKey(key);
}
public Command createRule(K sourceKey, K destKey, Aggregation aggregation, long timeBucket) {
notNull(sourceKey, "Source key");
notNull(destKey, "Destination key");
notNull(aggregation, "Aggregation");
CommandArgs args = args(sourceKey);
args.addKey(destKey);
args.add(CommandKeyword.AGGREGATION);
args.add(aggregation.getName());
args.add(timeBucket);
return createCommand(CommandType.CREATERULE, new StatusOutput<>(codec), args);
}
public Command deleteRule(K sourceKey, K destKey) {
notNull(sourceKey, "Source key");
notNull(destKey, "Destination key");
CommandArgs args = args(sourceKey);
args.addKey(destKey);
return createCommand(CommandType.DELETERULE, new StatusOutput<>(codec), args);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy