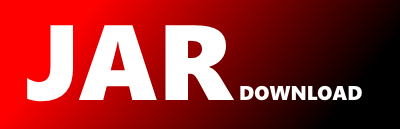
com.redislabs.picocliredis.Application Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of picocli-redis Show documentation
Show all versions of picocli-redis Show documentation
Picocli configuration for Redis
package com.redislabs.picocliredis;
import java.security.Security;
import java.util.logging.ConsoleHandler;
import java.util.logging.Level;
import java.util.logging.LogManager;
import java.util.logging.Logger;
import org.slf4j.LoggerFactory;
import io.netty.util.internal.logging.InternalLoggerFactory;
import io.netty.util.internal.logging.JdkLoggerFactory;
import lombok.Getter;
import lombok.Setter;
import picocli.CommandLine;
import picocli.CommandLine.ArgGroup;
import picocli.CommandLine.Command;
import picocli.CommandLine.Option;
import picocli.CommandLine.ParseResult;
import picocli.CommandLine.PicocliException;
@Command(abbreviateSynopsis = true, mixinStandardHelpOptions = true, versionProvider = ManifestVersionProvider.class, usageHelpAutoWidth = true, subcommands = HiddenGenerateCompletion.class, sortOptions = false)
public class Application implements Runnable {
private final static String DNS_CACHE_TTL = "networkaddress.cache.ttl";
private final static String DNS_CACHE_NEGATIVE_TTL = "networkaddress.cache.negative.ttl";
// private final static String SSL_PREFIX = "javax.net.ssl.";
// private final static String SSL_TRUST_STORE = SSL_PREFIX + "trustStore";
// private final static String SSL_TRUST_STORE_TYPE = SSL_PREFIX + "trustStoreType";
// private final static String SSL_TRUST_STORE_PASSWORD = SSL_PREFIX + "trustStorePassword";
// private final static String SSL_KEY_STORE = SSL_PREFIX + "keyStore";
// private final static String SSL_KEY_STORE_TYPE = SSL_PREFIX + "keyStoreType";
// private final static String SSL_KEY_STORE_PASSWORD = SSL_PREFIX + "keyStorePassword";
private static final String ROOT_LOGGER = "";
@Option(names = { "-v", "--verbose" }, description = "Enable verbose logging", order = 2)
private boolean verbose;
@Option(names = { "-d", "--debug" }, description = "Enable debug logging", order = 3)
private boolean debug;
@Option(names = { "-q", "--quiet" }, description = "Disable all logging", order = 1)
private boolean quiet;
@Option(names = "--dns-ttl", description = "DNS cache TTL", paramLabel = "", order = 4)
private int dnsTtl;
@Option(names = "--dns-neg-ttl", description = "DNS cache negative TTL", paramLabel = "", order = 4)
private int dnsNegativeTtl;
@CommandLine.Mixin
private @Getter @Setter RedisOptions redis = new RedisOptions();
public boolean isDebug() {
return debug;
}
public boolean isQuiet() {
return quiet;
}
public boolean isVerbose() {
return verbose;
}
public int execute(String... args) {
CommandLine commandLine = new CommandLine(this);
registerConverters(commandLine);
commandLine.setCaseInsensitiveEnumValuesAllowed(true);
try {
ParseResult parseResult = commandLine.parseArgs(args);
configureLogging();
configureDns();
return commandLine.getExecutionStrategy().execute(parseResult);
} catch (PicocliException e) {
System.err.println(e.getMessage());
return 1;
}
}
protected void registerConverters(CommandLine commandLine) {
commandLine.registerConverter(Server.class, s -> new Server(s));
}
private void configureDns() {
org.slf4j.Logger log = LoggerFactory.getLogger(getClass());
log.debug("Setting {}={}", DNS_CACHE_TTL, dnsTtl);
Security.setProperty(DNS_CACHE_TTL, String.valueOf(dnsTtl));
log.debug("Setting {}={}", DNS_CACHE_NEGATIVE_TTL, dnsNegativeTtl);
Security.setProperty(DNS_CACHE_NEGATIVE_TTL, String.valueOf(dnsNegativeTtl));
}
private void configureLogging() {
InternalLoggerFactory.setDefaultFactory(JdkLoggerFactory.INSTANCE);
LogManager.getLogManager().reset();
Logger activeLogger = Logger.getLogger(ROOT_LOGGER);
ConsoleHandler handler = new ConsoleHandler();
handler.setLevel(Level.ALL);
handler.setFormatter(new OneLineLogFormat(debug));
activeLogger.addHandler(handler);
Logger.getLogger(ROOT_LOGGER).setLevel(rootLoggingLevel());
Logger.getLogger(getClass().getPackage().getName()).setLevel(packageLoggingLevel());
}
private Level packageLoggingLevel() {
if (debug) {
return Level.FINEST;
}
if (verbose) {
return Level.FINE;
}
if (quiet) {
return Level.OFF;
}
return Level.INFO;
}
private Level rootLoggingLevel() {
if (debug) {
return Level.FINE;
}
if (verbose) {
return Level.INFO;
}
if (quiet) {
return Level.OFF;
}
return Level.SEVERE;
}
@Override
public void run() {
CommandLine.usage(this, System.out);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy