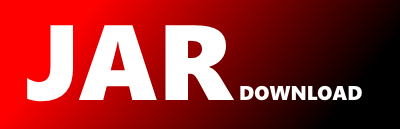
com.redislabs.riot.batch.processor.SpelProcessor Maven / Gradle / Ivy
package com.redislabs.riot.batch.processor;
import java.lang.reflect.Method;
import java.text.DateFormat;
import java.util.Arrays;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Map.Entry;
import org.springframework.batch.item.ItemProcessor;
import org.springframework.expression.Expression;
import org.springframework.expression.ExpressionInvocationTargetException;
import org.springframework.expression.spel.standard.SpelExpressionParser;
import org.springframework.expression.spel.support.StandardEvaluationContext;
import com.redislabs.riot.batch.MapAccessor;
import lombok.Getter;
import lombok.experimental.Accessors;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Accessors(fluent = true)
public class SpelProcessor implements ItemProcessor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy