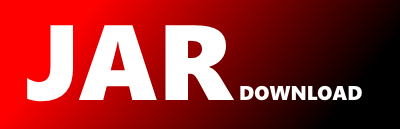
Net.5.0.Rosetta.Meta.MetaInterfaces.cs Maven / Gradle / Ivy
#nullable enable // Allow nullable reference types
namespace Rosetta.Lib.Meta
{
using System;
using System.Collections.Generic;
using Newtonsoft.Json;
using Rosetta.Lib.Validation;
public interface IFieldWithMeta where T : class
{
T Value { get; }
}
public interface IEnumFieldWithMeta where T : struct, System.Enum
{
T Value { get; }
}
public interface IValueFieldWithMeta where T : struct
{
T Value { get; }
}
public interface IFieldWithMetaBuilder
{
IFieldWithMetaBuilder SetValue(T value);
}
public interface IGlobalKeyFields
{
string GlobalKey { get; }
string ExternalKey { get; }
}
interface IGlobalKeyFieldsBuilder : IGlobalKeyFields
{
IGlobalKeyFieldsBuilder SetGlobalKey(string globalKey);
IGlobalKeyFieldsBuilder SetExternalKey(string externalKey);
}
public interface IMetaDataFields
{
string Scheme { get; }
}
interface IMetaDataFieldsBuilder : IMetaDataFields
{
IMetaDataFieldsBuilder SetScheme(string scheme);
}
public interface IReferenceWithMeta where T : class
{
string? GlobalReference { get; }
string? ExternalReference { get; }
T? Value { get; }
}
public interface IValueReferenceWithMeta where T : struct
{
string? GlobalReference { get; }
string? ExternalReference { get; }
T? Value { get; }
}
public interface IReferenceWithMetaBuilderBase
{
IReferenceWithMetaBuilderBase SetGlobalReference(string globalKey);
IReferenceWithMetaBuilderBase SetExternalReference(string ExternalKey);
string? GlobalReference { get; }
string? ExternalReference { get; }
//TODO: Type ValueType { get; }
}
public interface IReferenceWithMetaBuilder : IReferenceWithMetaBuilderBase
{
new IReferenceWithMetaBuilder SetGlobalReference(string globalKey);
new IReferenceWithMetaBuilder SetExternalReference(string ExternalKey);
//TODO: RosettaModelObjectBuilder getValue();
IReferenceWithMetaBuilder SetValue(I value);
}
public interface IBasicReferenceWithMetaBuilder : IReferenceWithMetaBuilderBase
{
new IBasicReferenceWithMetaBuilder SetGlobalReference(string globalKey);
new IBasicReferenceWithMetaBuilder SetExternalReference(string ExternalKey);
I Value { get; }
IBasicReferenceWithMetaBuilder SetValue(I value);
}
public interface IRosettaMetaData where T : IRosettaModelObject
{
[JsonIgnore]
IEnumerable> DataRules { get; }
[JsonIgnore]
IEnumerable> ChoiceRuleValidators { get; }
/*
[JsonIgnore]
TODO: IEnumerable> GetQualifyFunctions(QualifyFunctionFactory factory);
*/
[JsonIgnore]
IValidator Validator { get; }
[JsonIgnore]
IValidatorWithArg> OnlyExistsValidator { get; }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy