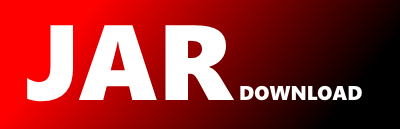
com.regnosys.rosetta.translate.util.JsonProcessor Maven / Gradle / Ivy
package com.regnosys.rosetta.translate.util;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.net.URL;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.SortedMap;
import java.util.TreeMap;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.regnosys.rosetta.common.util.UrlUtils;
/**
* Unintelligent pre-processor of ISDA CREATE JSON documents
*
* @author nikos
*/
public class JsonProcessor {
private final String baseNodeName;
private final ObjectMapper mapper;
public JsonProcessor(String baseNode, ObjectMapper mapper) {
this.baseNodeName = baseNode;
this.mapper = mapper;
}
/**
* Takes advantage of the fact that all likely work happens at one level
* When there is a partyA_xxx entry, ensure that there is a corresponding partyB_xxx entry
* and that partyA_xxx values come before partyB_xxx values
*
* @param input the JSON URL
* @return
* @throws IOException
*/
public Reader process(URL input) throws IOException {
return process(UrlUtils.openURL(input));
}
public Reader process(Reader input) throws IOException {
Map jsonMap = mapper.readValue(input, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy