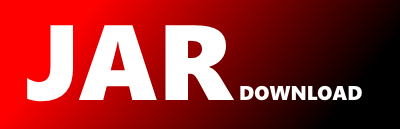
com.regnosys.rosetta.translate.HandlerCache Maven / Gradle / Ivy
package com.regnosys.rosetta.translate;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Supplier;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.collect.HashBasedTable;
import com.google.common.collect.Multimap;
import com.google.common.collect.Table;
import com.regnosys.rosetta.common.translation.Path;
import com.rosetta.model.lib.RosettaModelObjectBuilder;
/**
*
*
* @author TomForwood
* @param
* @param
*/
public class HandlerCache {
private static final Logger LOGGER = LoggerFactory.getLogger(HandlerCache.class);
/**
* A cache of the synonyms associated with each final attribute of the class associated with this cache
*/
private final Multimap synonyms;
/**
* The number of handlers that have been created for an attribute
*/
private final Map handlersPerAttributeCount;
/**
* A master list of the handlers in the same order as the underlying objects
*/
private final List> masterHandlerList;
/**
* A cache of attribute, synonymsGroup, cardinality -> handler
* (where cardinality is the index in the list)
*/
private final Table>> handlerCache;
public HandlerCache(Multimap synonyms) {
this.synonyms = synonyms;
handlersPerAttributeCount = new HashMap<>();
masterHandlerList = new ArrayList<>();
handlerCache = HashBasedTable.create();
}
public ROMParseHandler getOrCreateHandler(String attribute, Path xmlPath, Supplier extends ROMParseHandler> supplier){
Collection groups = synonyms.get(attribute);
SynonymValueGroup group = groups.stream().filter(g->g.startMatches(xmlPath)).findFirst().orElse(null);
if (group == null) {
LOGGER.debug("SynonymValueGroup not found for {}", xmlPath);
group = new SynonymValueGroup();
}
int cardinality = xmlPath.cardinality();
List> handlers = handlerCache.get(attribute, group);
if (handlers == null) {
handlers = new ArrayList<>();
handlerCache.put(attribute, group, handlers);
}
if (handlers.size()>cardinality) {
ROMParseHandler result = handlers.get(cardinality);
result.addXmlPath(xmlPath);
return result;
}
//get the number of handlers that have already been associated with this attribute
Integer handlerCount = handlersPerAttributeCount.merge(attribute, Integer.valueOf(0), (k,i)->k+1);
ROMParseHandler result=null;
if (handlerCount> getAllHandlers() {
return masterHandlerList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy