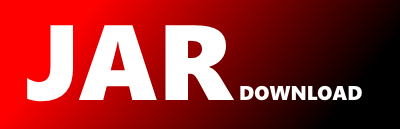
com.regnosys.rosetta.translate.datamodel.json.schema.JavaFileWriter Maven / Gradle / Ivy
The newest version!
package com.regnosys.rosetta.translate.datamodel.json.schema;
import com.regnosys.rosetta.translate.datamodel.Cardinality;
import com.rosetta.util.DottedPath;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
import org.apache.log4j.Logger;
import org.eclipse.xtend.lib.annotations.Data;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.Pure;
import org.eclipse.xtext.xbase.lib.StringExtensions;
import org.eclipse.xtext.xbase.lib.util.ToStringBuilder;
@SuppressWarnings("all")
public class JavaFileWriter {
@Data
public static class PojoClass {
private final String name;
private final List attributes;
public PojoClass(final String name, final List attributes) {
super();
this.name = name;
this.attributes = attributes;
}
@Override
@Pure
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((this.name== null) ? 0 : this.name.hashCode());
return prime * result + ((this.attributes== null) ? 0 : this.attributes.hashCode());
}
@Override
@Pure
public boolean equals(final Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
JavaFileWriter.PojoClass other = (JavaFileWriter.PojoClass) obj;
if (this.name == null) {
if (other.name != null)
return false;
} else if (!this.name.equals(other.name))
return false;
if (this.attributes == null) {
if (other.attributes != null)
return false;
} else if (!this.attributes.equals(other.attributes))
return false;
return true;
}
@Override
@Pure
public String toString() {
ToStringBuilder b = new ToStringBuilder(this);
b.add("name", this.name);
b.add("attributes", this.attributes);
return b.toString();
}
@Pure
public String getName() {
return this.name;
}
@Pure
public List getAttributes() {
return this.attributes;
}
}
@Data
public static class PojoAttribute {
private final String jsonProperty;
private final String name;
private final String className;
private final Cardinality cardinality;
public PojoAttribute(final String jsonProperty, final String name, final String className, final Cardinality cardinality) {
super();
this.jsonProperty = jsonProperty;
this.name = name;
this.className = className;
this.cardinality = cardinality;
}
@Override
@Pure
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((this.jsonProperty== null) ? 0 : this.jsonProperty.hashCode());
result = prime * result + ((this.name== null) ? 0 : this.name.hashCode());
result = prime * result + ((this.className== null) ? 0 : this.className.hashCode());
return prime * result + ((this.cardinality== null) ? 0 : this.cardinality.hashCode());
}
@Override
@Pure
public boolean equals(final Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
JavaFileWriter.PojoAttribute other = (JavaFileWriter.PojoAttribute) obj;
if (this.jsonProperty == null) {
if (other.jsonProperty != null)
return false;
} else if (!this.jsonProperty.equals(other.jsonProperty))
return false;
if (this.name == null) {
if (other.name != null)
return false;
} else if (!this.name.equals(other.name))
return false;
if (this.className == null) {
if (other.className != null)
return false;
} else if (!this.className.equals(other.className))
return false;
if (this.cardinality == null) {
if (other.cardinality != null)
return false;
} else if (!this.cardinality.equals(other.cardinality))
return false;
return true;
}
@Override
@Pure
public String toString() {
ToStringBuilder b = new ToStringBuilder(this);
b.add("jsonProperty", this.jsonProperty);
b.add("name", this.name);
b.add("className", this.className);
b.add("cardinality", this.cardinality);
return b.toString();
}
@Pure
public String getJsonProperty() {
return this.jsonProperty;
}
@Pure
public String getName() {
return this.name;
}
@Pure
public String getClassName() {
return this.className;
}
@Pure
public Cardinality getCardinality() {
return this.cardinality;
}
}
private static final Logger LOGGER = Logger.getLogger(JavaFileWriter.class);
public CharSequence toJava(final DottedPath mainPackage, final DottedPath sharedPackage, final JavaFileWriter.PojoClass c) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("package ");
_builder.append(mainPackage);
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("import com.fasterxml.jackson.annotation.JsonProperty;");
_builder.newLine();
{
boolean _containsList = this.containsList(c.attributes);
if (_containsList) {
_builder.append("import java.util.List;");
_builder.newLine();
}
}
{
if ((mainPackage != sharedPackage)) {
_builder.append("import ");
_builder.append(sharedPackage);
_builder.append(".*;");
_builder.newLineIfNotEmpty();
}
}
_builder.newLine();
_builder.append("public class ");
_builder.append(c.name);
_builder.append(" {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.newLine();
{
boolean _hasElements = false;
for(final JavaFileWriter.PojoAttribute a : c.attributes) {
if (!_hasElements) {
_hasElements = true;
} else {
_builder.appendImmediate("\n", "\t");
}
_builder.append("\t");
_builder.append("@JsonProperty(\"");
_builder.append(a.jsonProperty, "\t");
_builder.append("\")");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("private ");
String _firstUpper = StringExtensions.toFirstUpper(this.toAttributeClass(a));
_builder.append(_firstUpper, "\t");
_builder.append(" ");
_builder.append(a.name, "\t");
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
_builder.append("\t");
_builder.newLine();
{
boolean _hasElements_1 = false;
for(final JavaFileWriter.PojoAttribute a_1 : c.attributes) {
if (!_hasElements_1) {
_hasElements_1 = true;
} else {
_builder.appendImmediate("\n", "\t");
}
_builder.append("\t");
_builder.append("public ");
String _firstUpper_1 = StringExtensions.toFirstUpper(this.toAttributeClass(a_1));
_builder.append(_firstUpper_1, "\t");
_builder.append(" get");
String _firstUpper_2 = StringExtensions.toFirstUpper(a_1.name);
_builder.append(_firstUpper_2, "\t");
_builder.append("() {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t");
_builder.append("return ");
_builder.append(a_1.name, "\t\t");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.newLine();
_builder.append("\t");
_builder.append("public void set");
String _firstUpper_3 = StringExtensions.toFirstUpper(a_1.name);
_builder.append(_firstUpper_3, "\t");
_builder.append("(");
String _firstUpper_4 = StringExtensions.toFirstUpper(this.toAttributeClass(a_1));
_builder.append(_firstUpper_4, "\t");
_builder.append(" ");
_builder.append(a_1.name, "\t");
_builder.append(") {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t");
_builder.append("this.");
_builder.append(a_1.name, "\t\t");
_builder.append(" = ");
_builder.append(a_1.name, "\t\t");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("}");
_builder.newLine();
}
}
_builder.append("}");
_builder.newLine();
return _builder;
}
public void writeFile(final Path outputDir, final DottedPath mainPackage, final String fileName, final String contents) {
try {
final Path path = outputDir.resolve(mainPackage.toPath());
boolean _exists = Files.exists(path);
boolean _not = (!_exists);
if (_not) {
Files.createDirectories(path);
}
final Path fullPath = path.resolve((fileName + ".java"));
JavaFileWriter.LOGGER.debug(("Writing class " + fullPath));
Files.write(fullPath, contents.getBytes());
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
}
private String toAttributeClass(final JavaFileWriter.PojoAttribute a) {
if ((a.cardinality == Cardinality.MULTIPLE)) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("List<");
_builder.append(a.className);
_builder.append(">");
return _builder.toString();
} else {
return a.className;
}
}
private boolean containsList(final List attributes) {
final Function1 _function = (JavaFileWriter.PojoAttribute it) -> {
return Boolean.valueOf((it.cardinality == Cardinality.MULTIPLE));
};
return IterableExtensions.exists(attributes, _function);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy