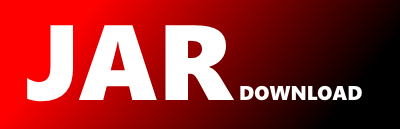
com.rei.ezup.index.TemplateIndex Maven / Gradle / Ivy
The newest version!
package com.rei.ezup.index;
import static java.util.stream.Collectors.toList;
import java.io.IOException;
import java.io.UncheckedIOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.stream.Stream;
import groovy.json.JsonSlurper;
import org.eclipse.aether.repository.RemoteRepository;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.rei.aether.Aether;
import com.rei.ezup.EzUp;
import com.rei.ezup.TemplateInfo;
public class TemplateIndex {
private static final Logger logger = LoggerFactory.getLogger(TemplateIndex.class);
public static final String EXTENSION = "ezup";
private static final String RELEASE_VERSION = ":RELEASE";
private static final String CENTRAL_SEARCH_URL =
"https://search.maven.org/solrsearch/select?q=p:" + EXTENSION + "&core=gav&rows=100&wt=json";
//TODO: implement nexus3 indexer
private static final List REMOTE_REPOSITORY_INDEXERS = Collections.emptyList();
private EzUp ezup;
private Aether aether;
private Map templates = new ConcurrentHashMap<>();
public TemplateIndex(EzUp ezup, Aether aether) {
this.ezup = ezup;
this.aether = aether;
}
public void store(Path file) throws IOException {
Files.write(file, templates.keySet());
}
public void load(Path file) throws IOException {
Files.readAllLines(file).forEach(this::index);
}
public void reindex() {
logger.info("performing reindex of known templates...");
Stream.of(
() -> reindexDirectory(aether.getLocalRepository().getBasedir().toPath()),
() -> aether.getConfiguredRepositories().parallelStream().forEach(this::index),
this::indexMavenCentral
).parallel().forEach(Runnable::run);
}
@SuppressWarnings("unchecked")
private List indexMavenCentral() {
try {
logger.info("indexing maven central...");
Map> result = (Map>) new JsonSlurper().parse(new URL(CENTRAL_SEARCH_URL));
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy