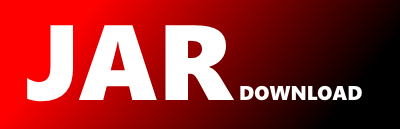
com.remondis.limbus.system.SystemConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of limbus-system Show documentation
Show all versions of limbus-system Show documentation
The Limbus System is a small light-weight CDI framework managing the Limbus Core Components.
The object graph is represented by an XML configuration file or can be build using the Limbus System API.
This module delivers an optional system component that visualizes the object graph and its dependencies after initializing: com.remondis.limbus.system.visualize.LimbusSystemVisualizer
This component can be added to the Limbus System. To keep the dependencies of this module transparent and light-weight, the graph renderer is declared as an optional dependency. Add the following dependencies to your project to use the visualisation component:
<!-- Graph Stream for Visualization feature This is an optional dependency and only required if using the com.remondis.limbus.system.visualize.LimbusSystemVisualizer -->
<dependency>
<groupId>org.graphstream</groupId>
<artifactId>gs-core</artifactId>
<version>1.3</version>
</dependency>
<dependency>
<groupId>org.graphstream</groupId>
<artifactId>gs-ui</artifactId>
<version>1.3</version>
</dependency>
package com.remondis.limbus.system;
import java.io.Serializable;
import java.util.LinkedList;
import java.util.List;
import com.remondis.limbus.IInitializable;
import com.remondis.limbus.Initializable;
import com.remondis.limbus.utils.Lang;
import com.remondis.limbus.utils.SerializeException;
import com.thoughtworks.xstream.annotations.XStreamAlias;
import com.thoughtworks.xstream.annotations.XStreamImplicit;
/**
* This class encapsulates the system configuration that references components to be build and specifies their
* (de)initialization order.
*
* @author schuettec
*
*/
@XStreamAlias(value = "SystemConfiguration", impl = SystemConfiguration.class)
final class SystemConfiguration implements Serializable {
/**
*
*/
private static final long serialVersionUID = 1L;
/**
* Holds the object factory that is to be used.
*/
protected ObjectFactory objectFactory;
@XStreamImplicit
public List components;
public static void main(String[] args) throws SerializeException {
SystemConfiguration conf = new SystemConfiguration();
conf.setObjectFactory(new ReflectiveObjectFactory());
conf.addComponentConfiguration(new ComponentConfiguration(Initializable.class, Initializable.class));
LimbusSystem.DEFAULT_XSTREAM.writeObject(conf, System.out);
}
/**
* Creates a new {@link SystemConfiguration} containig all component configurations from the specified configuration.
*
* @param configuration
* The configuration to copy.
*/
public SystemConfiguration(SystemConfiguration configuration) {
Lang.denyNull("configuration", configuration);
this.components = new LinkedList<>(configuration.components);
this.objectFactory = configuration.objectFactory;
}
public SystemConfiguration() {
readResolve();
}
protected Object readResolve() {
if (components == null) {
components = new LinkedList();
}
if (objectFactory == null) {
objectFactory = LimbusSystem.DEFAULT_FACTORY;
}
return this;
}
/**
* Adds a new component configuration to the system configuration.
*
* @param component
* The component configuration to add.
*/
public void addComponentConfiguration(ComponentConfiguration component) {
Lang.denyNull("component", component);
// schuettec - 27.03.2017 : Due to the fact that the Limbus System mocking API must be able to replace
// ComponentConfigurations, we have to update existing configurations!
if (components.contains(component)) {
components.remove(component);
}
components.add(component);
}
public boolean containsComponentConfiguration(ComponentConfiguration component) {
Lang.denyNull("component", component);
return components.contains(component);
}
/**
* Adds a new component configuration to the system configuration.
*
* @param component
* The component configuration to add.
*/
public void removeComponentConfiguration(ComponentConfiguration component) {
Lang.denyNull("component", component);
if (components.contains(component)) {
components.remove(component);
}
}
/**
* Removes a component configuration by request type.
*/
> void removeByRequestType(Class requestType) {
Lang.denyNull("requestType", requestType);
ComponentConfiguration conf = new ComponentConfiguration(requestType, null);
components.remove(conf);
}
/**
* @return the objectFactory
*/
public ObjectFactory getObjectFactory() {
return objectFactory;
}
/**
* @param objectFactory
* the objectFactory to set
*/
public void setObjectFactory(ObjectFactory objectFactory) {
this.objectFactory = objectFactory;
}
/**
* @return the components Returns a new list containig the configured components.
*/
List getComponents() {
return new LinkedList<>(components);
}
/**
* Checks if the specified request type is already added to the {@link SystemConfiguration}.
*
* @param requestType
* The request type to check for.
* @return Returns true
if the specified request type is already added to the {@link SystemConfiguration}
* , otherwise false
is returned.
*/
public > boolean containsRequestType(Class requestType) {
Lang.denyNull("requestType", requestType);
return containsComponentConfiguration(new ComponentConfiguration(requestType, null));
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder b = new StringBuilder("System Configuration\n");
for (ComponentConfiguration c : components) {
b.append(c.toString())
.append("\n");
}
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy