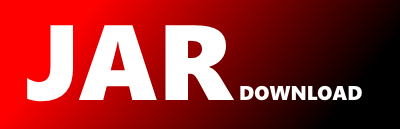
com.reprezen.genflow.common.doc.XDocHelper Maven / Gradle / Ivy
/**
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*/
package com.reprezen.genflow.common.doc;
import com.reprezen.rapidml.Constraint;
import com.reprezen.rapidml.DataModel;
import com.reprezen.rapidml.Documentable;
import com.reprezen.rapidml.Documentation;
import com.reprezen.rapidml.Feature;
import com.reprezen.rapidml.LengthConstraint;
import com.reprezen.rapidml.RegExConstraint;
import com.reprezen.rapidml.ResourceAPI;
import com.reprezen.rapidml.ValueRangeConstraint;
import com.reprezen.rapidml.ZenModel;
import java.io.File;
import java.net.URI;
import java.util.Collections;
import java.util.List;
import java.util.regex.Pattern;
import org.apache.commons.text.StringEscapeUtils;
import org.commonmark.Extension;
import org.commonmark.ext.autolink.AutolinkExtension;
import org.commonmark.ext.gfm.tables.TablesExtension;
import org.commonmark.parser.Parser;
import org.commonmark.renderer.html.HtmlRenderer;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.util.EcoreUtil;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Exceptions;
@SuppressWarnings("all")
public class XDocHelper {
private static final List extensions = Collections.unmodifiableList(CollectionLiterals.newArrayList(TablesExtension.create(), AutolinkExtension.create()));
private static final Parser parser = Parser.builder().extensions(XDocHelper.extensions).build();
private static final HtmlRenderer htmlRenderer = HtmlRenderer.builder().extensions(XDocHelper.extensions).build();
private final URI baseUri;
public XDocHelper(final URI baseUri) {
this.baseUri = baseUri;
}
public String generateDoc(final Documentable documentable) {
Documentation _documentation = null;
if (documentable!=null) {
_documentation=documentable.getDocumentation();
}
String _text = null;
if (_documentation!=null) {
_text=_documentation.getText();
}
String _generateDoc = null;
if (_text!=null) {
_generateDoc=this.generateDoc(_text);
}
return _generateDoc;
}
public String generateDoc(final String text) {
String _markdown = null;
if (text!=null) {
_markdown=this.markdown(text);
}
return _markdown;
}
private String markdown(final String text) {
String _xblockexpression = null;
{
final String html = XDocHelper.htmlRenderer.render(XDocHelper.parser.parse(this.preprocess(text))).toString();
_xblockexpression = html.replaceAll("", "");
}
return _xblockexpression;
}
private static final Pattern NON_DOT_LINE = Pattern.compile("(?m)^[^.]");
private String preprocess(final String text) {
String _xifexpression = null;
boolean _find = XDocHelper.NON_DOT_LINE.matcher(text).find();
if (_find) {
_xifexpression = text;
} else {
_xifexpression = text.replaceAll("(?m)^[.]", "");
}
return _xifexpression;
}
public String htmlEscape(final String value) {
return StringEscapeUtils.escapeHtml4(value);
}
public CharSequence generateDocItem(final String text) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
String _generateDoc = this.generateDoc(text);
_builder.append(_generateDoc);
_builder.append("");
return _builder;
}
public CharSequence generateDocItem(final Documentable documentable) {
Documentation _documentation = null;
if (documentable!=null) {
_documentation=documentable.getDocumentation();
}
String _text = null;
if (_documentation!=null) {
_text=_documentation.getText();
}
CharSequence _generateDocItem = null;
if (_text!=null) {
_generateDocItem=this.generateDocItem(_text);
}
return _generateDocItem;
}
public String htmlLink(final EObject obj) {
try {
final org.eclipse.emf.common.util.URI objUri = EcoreUtil.getURI(obj);
final String fileString = objUri.toFileString();
Object _xifexpression = null;
if ((fileString != null)) {
URI _xblockexpression = null;
{
URI rel = this.baseUri.relativize(new File(fileString).toURI());
String _scheme = rel.getScheme();
String _schemeSpecificPart = rel.getSchemeSpecificPart();
String _fragment = objUri.fragment();
_xblockexpression = new URI(_scheme, _schemeSpecificPart, _fragment);
}
_xifexpression = _xblockexpression;
} else {
_xifexpression = objUri;
}
Object fixedUri = _xifexpression;
String _sanitizeLink = this.sanitizeLink(fixedUri.toString());
return ("anchor:" + _sanitizeLink);
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
}
private String sanitizeLink(final String link) {
return link.replaceAll("[^a-zA-Z0-9_]", "_");
}
public String nameOrTitle(final ZenModel element) {
String _elvis = null;
String _title = element.getTitle();
if (_title != null) {
_elvis = _title;
} else {
String _name = element.getName();
_elvis = _name;
}
return _elvis;
}
public String nameOrTitle(final ResourceAPI element) {
String _elvis = null;
String _title = element.getTitle();
if (_title != null) {
_elvis = _title;
} else {
String _name = element.getName();
_elvis = _name;
}
return _elvis;
}
public String nameOrTitle(final DataModel element) {
String _elvis = null;
String _title = element.getTitle();
if (_title != null) {
_elvis = _title;
} else {
String _name = element.getName();
_elvis = _name;
}
return _elvis;
}
/**
* Data type properties are shown as rows in a table, elements that don't have their own anchor. Therefore, reusing the link of the containing data type
*/
public String htmlLinkReferenceProp(final Feature feature) {
return this.htmlLink(feature.getContainingDataType());
}
public CharSequence generateInlineConstraints(final List constraints) {
StringConcatenation _builder = new StringConcatenation();
{
boolean _isEmpty = constraints.isEmpty();
boolean _not = (!_isEmpty);
if (_not) {
_builder.append("");
_builder.newLine();
_builder.append("\t");
_builder.append("");
_builder.newLine();
_builder.append("\t\t");
_builder.append("Constraints ");
_builder.newLine();
_builder.append("\t");
_builder.append(" ");
_builder.newLine();
{
for(final Constraint constraint : constraints) {
_builder.append("");
_builder.newLine();
_builder.append("\t");
_builder.append("");
String _constraintType = this.getConstraintType(constraint);
_builder.append(_constraintType, "\t");
_builder.append(" ");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("");
CharSequence _constraintValue = this.getConstraintValue(constraint);
_builder.append(_constraintValue, "\t");
_builder.append(" ");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
}
}
_builder.append("
");
_builder.newLine();
}
}
return _builder;
}
public CharSequence generateConstraints(final List constraints) {
StringConcatenation _builder = new StringConcatenation();
{
boolean _isEmpty = constraints.isEmpty();
boolean _not = (!_isEmpty);
if (_not) {
_builder.append("Constraints
");
_builder.newLine();
_builder.append("");
_builder.newLine();
{
for(final Constraint constraint : constraints) {
_builder.append("");
_builder.newLine();
_builder.append("\t");
_builder.append("");
String _constraintType = this.getConstraintType(constraint);
_builder.append(_constraintType, "\t");
_builder.append(" ");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("");
CharSequence _constraintValue = this.getConstraintValue(constraint);
_builder.append(_constraintValue, "\t");
_builder.append(" ");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
}
}
_builder.append("
");
_builder.newLine();
}
}
return _builder;
}
public String getConstraintType(final Constraint constraint) {
String _switchResult = null;
boolean _matched = false;
if (constraint instanceof LengthConstraint) {
_matched=true;
_switchResult = "String Length";
}
if (!_matched) {
if (constraint instanceof RegExConstraint) {
_matched=true;
_switchResult = "Regular Expression";
}
}
if (!_matched) {
if (constraint instanceof ValueRangeConstraint) {
_matched=true;
_switchResult = "Value Range";
}
}
if (!_matched) {
_matched=true;
_switchResult = "";
}
return _switchResult;
}
public CharSequence getConstraintValue(final Constraint constraint) {
CharSequence _switchResult = null;
boolean _matched = false;
if (constraint instanceof LengthConstraint) {
_matched=true;
String _xifexpression = null;
boolean _isSetMinLength = ((LengthConstraint)constraint).isSetMinLength();
if (_isSetMinLength) {
String _xifexpression_1 = null;
boolean _isSetMaxLength = ((LengthConstraint)constraint).isSetMaxLength();
boolean _not = (!_isSetMaxLength);
if (_not) {
_xifexpression_1 = "minimum ";
} else {
_xifexpression_1 = "";
}
String _plus = ("from " + _xifexpression_1);
int _minLength = ((LengthConstraint)constraint).getMinLength();
String _plus_1 = (_plus + Integer.valueOf(_minLength));
String _xifexpression_2 = null;
boolean _isSetMaxLength_1 = ((LengthConstraint)constraint).isSetMaxLength();
if (_isSetMaxLength_1) {
int _maxLength = ((LengthConstraint)constraint).getMaxLength();
_xifexpression_2 = (" to " + Integer.valueOf(_maxLength));
} else {
_xifexpression_2 = "";
}
_xifexpression = (_plus_1 + _xifexpression_2);
} else {
int _maxLength_1 = ((LengthConstraint)constraint).getMaxLength();
_xifexpression = ("up to " + Integer.valueOf(_maxLength_1));
}
_switchResult = _xifexpression;
}
if (!_matched) {
if (constraint instanceof RegExConstraint) {
_matched=true;
StringConcatenation _builder = new StringConcatenation();
_builder.append("matching regex \"");
String _pattern = ((RegExConstraint)constraint).getPattern();
_builder.append(_pattern);
_builder.append("\"");
_switchResult = _builder;
}
}
if (!_matched) {
if (constraint instanceof ValueRangeConstraint) {
_matched=true;
String _xblockexpression = null;
{
String _xifexpression = null;
if ((((ValueRangeConstraint)constraint).isMinValueExclusive() || ((ValueRangeConstraint)constraint).isMaxValueExclusive())) {
String _xifexpression_1 = null;
boolean _isMaxValueExclusive = ((ValueRangeConstraint)constraint).isMaxValueExclusive();
if (_isMaxValueExclusive) {
_xifexpression_1 = " exclusive";
} else {
_xifexpression_1 = " inclusive";
}
_xifexpression = _xifexpression_1;
} else {
_xifexpression = "";
}
final String maxExcStr = _xifexpression;
String _xifexpression_2 = null;
if ((((ValueRangeConstraint)constraint).isMinValueExclusive() || ((ValueRangeConstraint)constraint).isMaxValueExclusive())) {
String _xifexpression_3 = null;
boolean _isMinValueExclusive = ((ValueRangeConstraint)constraint).isMinValueExclusive();
if (_isMinValueExclusive) {
_xifexpression_3 = " exclusive";
} else {
_xifexpression_3 = " inclusive";
}
_xifexpression_2 = _xifexpression_3;
} else {
_xifexpression_2 = "";
}
final String minExcStr = _xifexpression_2;
String _xifexpression_4 = null;
String _minValue = ((ValueRangeConstraint)constraint).getMinValue();
boolean _tripleNotEquals = (_minValue != null);
if (_tripleNotEquals) {
String _xifexpression_5 = null;
String _maxValue = ((ValueRangeConstraint)constraint).getMaxValue();
boolean _tripleEquals = (_maxValue == null);
if (_tripleEquals) {
_xifexpression_5 = "minimum ";
} else {
_xifexpression_5 = "";
}
String _plus = ("from " + _xifexpression_5);
String _minValue_1 = ((ValueRangeConstraint)constraint).getMinValue();
String _plus_1 = (_plus + _minValue_1);
String _plus_2 = (_plus_1 + minExcStr);
String _xifexpression_6 = null;
String _maxValue_1 = ((ValueRangeConstraint)constraint).getMaxValue();
boolean _tripleNotEquals_1 = (_maxValue_1 != null);
if (_tripleNotEquals_1) {
String _maxValue_2 = ((ValueRangeConstraint)constraint).getMaxValue();
String _plus_3 = (" to " + _maxValue_2);
_xifexpression_6 = (_plus_3 + maxExcStr);
} else {
_xifexpression_6 = "";
}
_xifexpression_4 = (_plus_2 + _xifexpression_6);
} else {
String _maxValue_3 = ((ValueRangeConstraint)constraint).getMaxValue();
String _plus_4 = ("up to" + _maxValue_3);
_xifexpression_4 = (_plus_4 + maxExcStr);
}
_xblockexpression = _xifexpression_4;
}
_switchResult = _xblockexpression;
}
}
if (!_matched) {
_matched=true;
_switchResult = "";
}
return _switchResult;
}
public static CharSequence tableWithHeader(final String tableName, final String... columns) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.append(tableName);
_builder.append("
");
_builder.newLineIfNotEmpty();
_builder.append("");
_builder.newLine();
_builder.append(" ");
_builder.append("");
_builder.newLine();
{
for(final String column : columns) {
_builder.append(" ");
_builder.append("");
_builder.append(column, " ");
_builder.append(" ");
_builder.newLineIfNotEmpty();
}
}
_builder.append(" ");
_builder.append(" ");
_builder.newLine();
return _builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy